Testing web applications is crucial for ensuring they function correctly and provide a good user experience. One of the fundamental tests is verifying that users can log in successfully. Here, I’ll demonstrate how to write a Cypress test to validate the login functionality of the SAP Hybris backoffice application. We’ll also explore two different approaches to handling the presence of a loading spinner that can interfere with the test.
Application Under Test
The application we are testing is the SAP Hybris backoffice login page, accessible at the following URL: https://backoffice.iqos.com/backoffice/login.zul
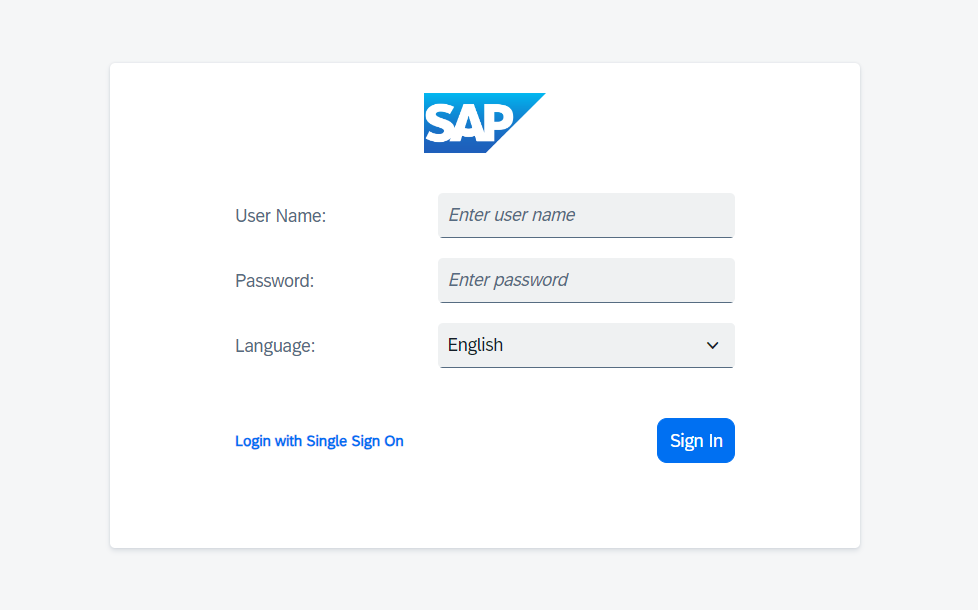
On this page, there are three key elements we will interact with:
- User name input field
- Password input field
- Sign in button
Here are the HTML codes for these elements:
<input id="fCZEv" class="login z-textbox" autocomplete="off" value="" type="text" name="j_username" placeholder="Enter user name" aria-disabled="false" aria-readonly="false">
<input id="fCZE_0" class="login z-textbox" autocomplete="off" value="" type="password" name="j_password" placeholder="Enter password" aria-disabled="false" aria-readonly="false">
<button type="button" id="fCZEo" class="login_btn y-btn-primary z-button">Sign In</button>
The Challenge
The primary challenge when testing this login functionality is dealing with a “Processing” spinner and an opaque mask (div.z-modal-mask
) that covers the input fields during the initial page load. The test will fail if we try to interact with the input fields while they are covered.
Solution 1: Waiting for the Spinner and Mask to Disappear
The first solution involves removing the spinner and mask before interacting with the input fields. This ensures that the elements are visible and interactable.
Test Code
Here’s the test code for the first solution:
cy.visit('https://backoffice.iqos.com/backoffice/login.zul');
cy.get('div.z-modal-mask') // Wait for the mask to appear
cy.get('div.z-modal-mask', { timeout: 10000 })
.should('not.exist'); // Wait for the mask to disappear
cy.get('input[placeholder="Enter user name"]')
.type("username");
cy.get('input[placeholder="Enter password"]')
.type("password");
cy.get('button.login_btn')
.click();
Explanation
- Visit the Page: We start by visiting the login page using
cy.visit
. - Wait for the Mask to Appear: We wait for the
div.z-modal-mask
to appear on the page. - Wait for the Mask to Disappear: We use a timeout to wait up to 10 seconds for the mask to be removed. This ensures that the input fields are not covered.
- Type Credentials: Once the mask is gone, we type the username and password into the respective input fields.
- Click Sign In: Finally, we click the “Sign In” button to submit the login form.
This approach ensures that the test interacts with the elements only when they are visible and ready for user input.
Solution 2: Using Visible Selectors
The second solution focuses on ensuring that we interact with visible elements, using the :visible
pseudo-class. This approach also handles the case where elements might still be covered by the mask or spinner.
Test Code
Here’s the test code for the second solution:
describe('Write into input element', () => {
it('should fill the input elements and sign in', () => {
// Visit the page where the input elements are located
cy.visit('https://backoffice.iqos.com/backoffice/login.zul');
cy.get('input[placeholder="Enter user name"]:visible')
.type("yourUsername");
cy.get('input[placeholder="Enter password"]:visible')
.type("yourPass");
// Click the sign-in button using its class or text
cy.get('.login_btn').click();
// alternatively we may use: cy.get('button:visible').contains("Sign In").click();
});
});
Explanation
- Describe the Test: We wrap our test in a
describe
block for better organization. - Visit the Page: Again, we start by visiting the login page.
- Type Credentials: We use
:visible
to ensure that we only interact with visible input fields. This avoids the issue of elements being covered. - Click Sign In: We locate and click the “Sign In” button using either its class or its text, ensuring it is visible.
This approach ensures we only interact with currently visible and interactable elements.
Culmination
Both solutions provided here aim to ensure the reliability and robustness of the login test for the SAP Hybris backoffice application.
- Solution 1: Wait for the loading spinner and mask to disappear before interacting with the input fields. This ensures the fields are not covered and are ready for user input.
- Solution 2: Uses visible selectors to interact only with elements currently visible on the page. This avoids the issue of covered elements.
Choosing between these solutions depends on your application’s specific behavior and personal preference. Both approaches ensure the test mimics real user interactions and provides reliable results.
Following these approaches ensures that your login tests are robust and resilient to common issues like loading spinners and overlay masks.