I have recently done some testing of the product replacement feature inside of SAP Hybris with Cypress. Soon after that I was asked to try to do the same test in another testing automation software called Playwright. I’ve been using Playwright since the beginning of this year.
You may check out the Playwright test code I’ve come up with here: https://github.com/NoToolsNoCraft/SAP-Hybris-Cypress-Test/blob/main/PlaywrightReplacementMatrixTest
This code was made based on the 3rd version of the Cypress code I’ve previously created. Basically, it is the same test following the same process, just with Playwright commands instead of the ones used in Cypress.
How to use this test script?
Change the market and the environment labels based on your needs:
// Define a constant for the catalog label
const CATALOG_LABEL = 'Tunisia Product Catalog : Staged';
In my example, I’ve used Tunisia as my market. You can replace it with any other country that you want to test. Also, you should change the environment label based on your needs (Staged or Online in this case).
This test doesn’t depend on the total time
// Increase timeout for each test if needed, since these tests doesn't depend on max time
test.setTimeout(600000);
Since this test doesn’t really depend on the max time, you may set it as much as is needed to finish your test or to check all listed products. In my test case, I used 6 minutes. You shouldn’t really change it unless you search for so many products that you get the timeout exceeded error. In that case, you just have to set more total time. No other .timeout should be changed in the code because they are set to match the SAP Hybris workflow.
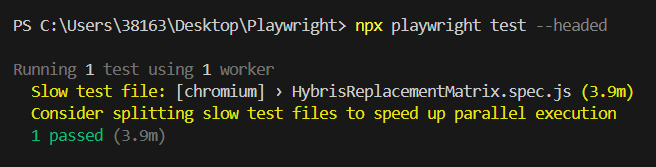
Modify the Hybris link
// Visit the login page before each test
await page.goto('https://backoffice.stg.iqos.com/backoffice/login.zul');
The Hybris link should be modified based on whether we are testing in the STG or PROD environment.
Enter your username and password
// Ensure the username field is visible and then fill it
await page.waitForSelector('input[placeholder="Enter user name"]:visible', { timeout: 10000 });
await page.fill('input[placeholder="Enter user name"]:visible', 'Enter your username');
// Ensure the password field is visible and then fill it
await page.waitForSelector('input[placeholder="Enter password"]:visible', { timeout: 10000 });
await page.fill('input[placeholder="Enter password"]:visible', 'Enter your password');
Modify product codes for product replacement test
Set the product code you want to search for:
await checkProductReplacements(page, "G0000605", [
{ code: "G0000605", level: 'L1' },
{ code: "G0000607", level: 'L1' },
{ code: "G0000608", level: 'L1' },
{ code: "G0000604", level: 'L1' },
{ code: "G0000592", level: 'L2' }
], true);
Set the codes of all products that should be available for replacement:
await checkProductReplacements(page, "G0000605", [
{ code: "G0000605", level: 'L1' },
{ code: "G0000607", level: 'L1' },
{ code: "G0000608", level: 'L1' },
{ code: "G0000604", level: 'L1' },
{ code: "G0000592", level: 'L2' }
], true);
Set the level for each product (L1, L2 or L3):
await checkProductReplacements(page, "G0000605", [
{ code: "G0000605", level: 'L1' },
{ code: "G0000607", level: 'L1' },
{ code: "G0000608", level: 'L1' },
{ code: "G0000604", level: 'L1' },
{ code: "G0000592", level: 'L2' }
], true);
You may add more or fewer products for replacement based on the requirement.
How do I run the test script?
To get the best tester-friendly experience, I’m using Virtual Studio Code. Through the terminal, I run the code by using this command:
npx playwright test --headed
This way, I’m opening the test in the previously chosen browsers—Google Chrome in my case. From my experience, this seems to be the best way to use this test script since we are simulating the same process as if someone would test the same features manually.