Recently, I had to validate the average ratings of products listed in an API response. Each product’s rating was crucial to ensure the accuracy of customer feedback, and I wanted to automate the process using Postman. In this article, I’ll walk you through the steps I took, explain the structure of the API response, and share the Postman script that helped me validate this data.
API Response Body
The API I was working with returned the following JSON structure for product details:
{
"Limit": 5,
"Offset": 0,
"TotalResults": 5,
"Locale": "en_US",
"Results": [
{
"ProductStatistics": {
"ProductId": "IQOSILUMAPrimeStarterKit",
"NativeReviewStatistics": {},
"ReviewStatistics": {
"AverageOverallRating": 4.7119,
"TotalReviewCount": 59,
"OverallRatingRange": 5
},
"QAStatistics": {}
}
},
{
"ProductStatistics": {
"ProductId": "IQOSILUMAStarterKit",
"NativeReviewStatistics": {},
"ReviewStatistics": {
"AverageOverallRating": 4.5714,
"TotalReviewCount": 105,
"OverallRatingRange": 5
},
"QAStatistics": {}
}
},
{
"ProductStatistics": {
"ProductId": "ILUMAONENeonStarterKit",
"NativeReviewStatistics": {},
"ReviewStatistics": {
"AverageOverallRating": 5.0,
"TotalReviewCount": 9,
"OverallRatingRange": 5
},
"QAStatistics": {}
}
},
{
"ProductStatistics": {
"ProductId": "IQOSILUMAOneStarterKit",
"NativeReviewStatistics": {},
"ReviewStatistics": {
"AverageOverallRating": 4.6025,
"TotalReviewCount": 161,
"OverallRatingRange": 5
},
"QAStatistics": {}
}
},
{
"ProductStatistics": {
"ProductId": "ILUMANeonStarterKit",
"NativeReviewStatistics": {},
"ReviewStatistics": {
"AverageOverallRating": 4.9333,
"TotalReviewCount": 15,
"OverallRatingRange": 5
},
"QAStatistics": {}
}
}
],
"Includes": {},
"HasErrors": false,
"Errors": []
}
What We Are Looking For
In the response above, each product has a ProductStatistics
object, which contains a ReviewStatistics
object. This ReviewStatistics
object holds information such as the AverageOverallRating
, the TotalReviewCount
, and the OverallRatingRange
for each product.
To ensure the data is valid:
ReviewStatistics
must exist: Each product should have aReviewStatistics
object, which contains the relevant review data.AverageOverallRating
must exist: Each product should have a validAverageOverallRating
field.AverageOverallRating
should be within the range: The rating should be between0
andOverallRatingRange
(which is5
in this case).
Postman Test Script
Here’s the Postman test script I used to validate the AverageOverallRating
for each product in the API response:
// Parse the JSON response
let jsonData = pm.response.json();
// Check if the Results array exists and is an array
pm.test("Results array exists", function () {
pm.expect(jsonData).to.have.property('Results');
pm.expect(jsonData.Results).to.be.an('array');
});
// Loop through each product in the Results array
jsonData.Results.forEach((product, index) => {
pm.test(`Product ${index + 1}: ReviewStatistics exists`, function () {
pm.expect(product.ProductStatistics).to.have.property('ReviewStatistics');
});
// Proceed only if ReviewStatistics exists
if (product.ProductStatistics.ReviewStatistics) {
pm.test(`Product ${index + 1}: AverageOverallRating exists`, function () {
pm.expect(product.ProductStatistics.ReviewStatistics).to.have.property('AverageOverallRating');
});
pm.test(`Product ${index + 1}: AverageOverallRating is a valid number`, function () {
let rating = product.ProductStatistics.ReviewStatistics.AverageOverallRating;
pm.expect(rating).to.be.a('number');
pm.expect(rating).to.be.within(0, product.ProductStatistics.ReviewStatistics.OverallRatingRange);
});
} else {
pm.test(`Product ${index + 1}: ReviewStatistics missing, skipping rating validation`, function () {
pm.expect(product.ProductStatistics.ReviewStatistics).to.be.undefined;
});
}
});
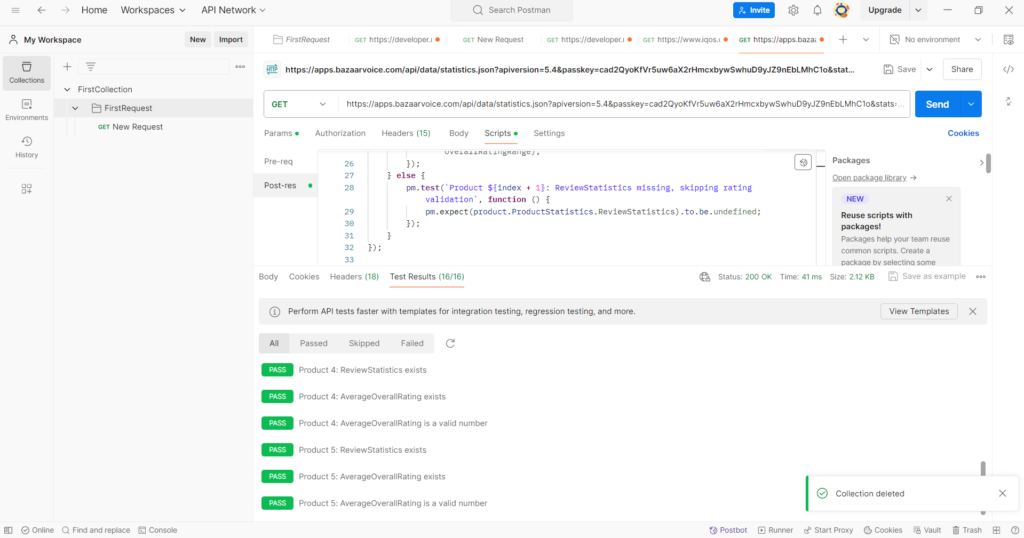
Detailed Explanation of the Script
- Parse the JSON Response: The first step is to parse the JSON response from the API using
pm.response.json()
. This converts the raw API response into a JavaScript object that can be easily navigated.let jsonData = pm.response.json();
- Check if the Results Array Exists: Since the products are stored in the
Results
array, I start by checking if theResults
array exists and if it’s an actual array.pm.test("Results array exists", function () { pm.expect(jsonData).to.have.property('Results'); pm.expect(jsonData.Results).to.be.an('array'); });
- Loop Through Each Product: I use the
forEach
method to loop through every product in theResults
array. For each product, the first thing I check is whetherReviewStatistics
exists.jsonData.Results.forEach((product, index) => { pm.test(`Product ${index + 1}: ReviewStatistics exists`, function () { pm.expect(product.ProductStatistics).to.have.property('ReviewStatistics'); }); });
- Check for
AverageOverallRating
: If theReviewStatistics
object exists, I check thatAverageOverallRating
is present and that it’s a valid number.pm.test(`Product ${index + 1}: AverageOverallRating exists`, function () { pm.expect(product.ProductStatistics.ReviewStatistics).to.have.property('AverageOverallRating'); });
- Validate the Rating: Finally, I validate that the rating is a number and that it falls within the correct range (between
0
andOverallRatingRange
).pm.test(`Product ${index + 1}: AverageOverallRating is a valid number`, function () { let rating = product.ProductStatistics.ReviewStatistics.AverageOverallRating; pm.expect(rating).to.be.a('number'); pm.expect(rating).to.be.within(0, product.ProductStatistics.ReviewStatistics.OverallRatingRange); });
- Handle Missing ReviewStatistics: If a product doesn’t have a
ReviewStatistics
object, the script skips the rating validation for that product and logs a message indicating the absence.pm.test(`Product ${index + 1}: ReviewStatistics missing, skipping rating validation`, function () { pm.expect(product.ProductStatistics.ReviewStatistics).to.be.undefined; });
Final Thoughts
By running this script, I automatically validated the Average Overall Rating of every product returned by the API. This saved me time and ensured consistent testing across various products. If you’re working with APIs and need to validate numerical values like ratings, this approach can help streamline your testing process.