Error message test
Here is the body text we received by running a GET request:
{
"Error": [
"BusinessUnit does not have access to that trustbox"
]
}
Here are some stuff that we could test:
- The script first checks if the status code is 400.
- Then, it ensures the response has an
Error
field. - Finally, it verifies that the specific error message
"BusinessUnit does not have access to that trustbox"
exists in theError
array.
This script perfectly does the requested job:
// Parse the response body
let responseData = pm.response.json();
// Check if the status code is 400 (or replace with the correct expected status code)
pm.test("Status code is 400", function () {
pm.response.to.have.status(400);
});
// Verify that the response contains the 'Error' field
pm.test("Response contains 'Error' field", function () {
pm.expect(responseData).to.have.property('Error');
});
// Check that the 'Error' array contains the specific message
pm.test("'BusinessUnit does not have access to that trustbox' error message is present", function () {
pm.expect(responseData.Error).to.include("BusinessUnit does not have access to that trustbox");
});
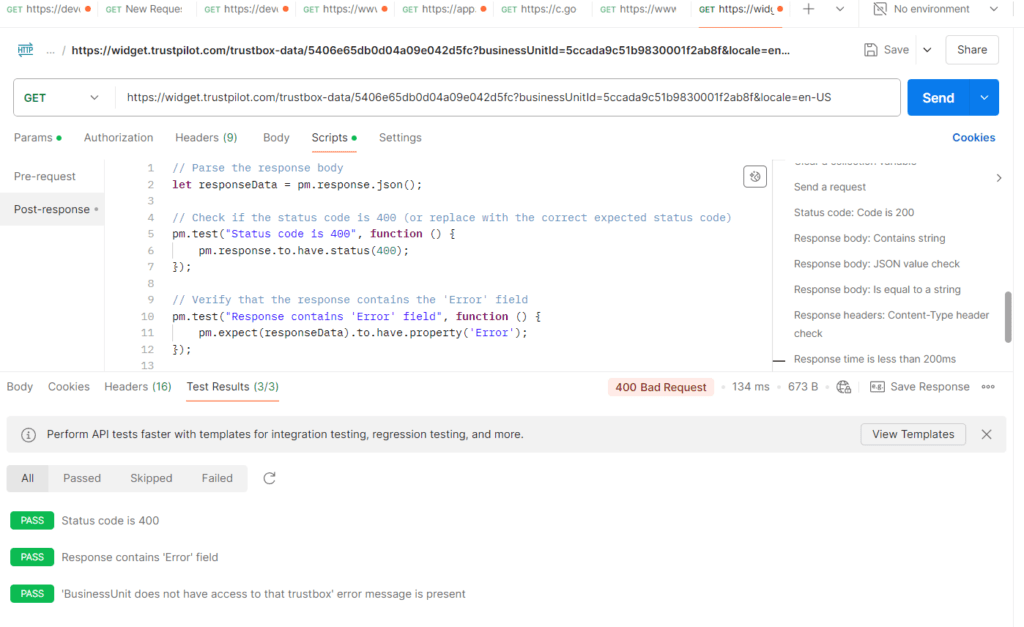
URL_Response_Testing
Here is the body text we received by running a GET request:
{
"url": "https://ir.ebaystatic.com/cr/v/c01/ac-090424201806.mweb.min.js",
"activeFactors": {}
}
Here’s the list of items tested by the Postman script:
- Status Code: Ensures the response returns a 200 (successful request) status code.
- Content-Type: Verifies that the
Content-Type
header of the response isapplication/json
, confirming that the response is a JavaScript file. - Non-Empty Response Body: Checks that the response body is not empty.
- JavaScript File Length: Ensures that the JSON file has a minimum length (can be adjusted as needed) to validate that the file contains actual content.
- Expected Script Content: Confirms that the response body contains specific expected script content (e.g., function names or important code segments).
Here is the testing script:
// Set up the request
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
// Check the content-type header
pm.test("Content-Type is application/json", function () {
pm.response.to.have.header("Content-Type", "application/json; charset=utf-8");
});
// Validate that the response body is not empty
pm.test("Response body is not empty", function () {
pm.response.to.not.be.empty;
});
// Validate that the JSON response has a specific structure or key
pm.test("Response contains 'url' key", function () {
var jsonData = pm.response.json();
pm.expect(jsonData).to.have.property("url");
});
// Check if the 'url' matches the expected URL
pm.test("URL matches expected value", function () {
var jsonData = pm.response.json();
pm.expect(jsonData.url).to.eql("https://ir.ebaystatic.com/cr/v/c01/ac-090424201806.mweb.min.js");
});
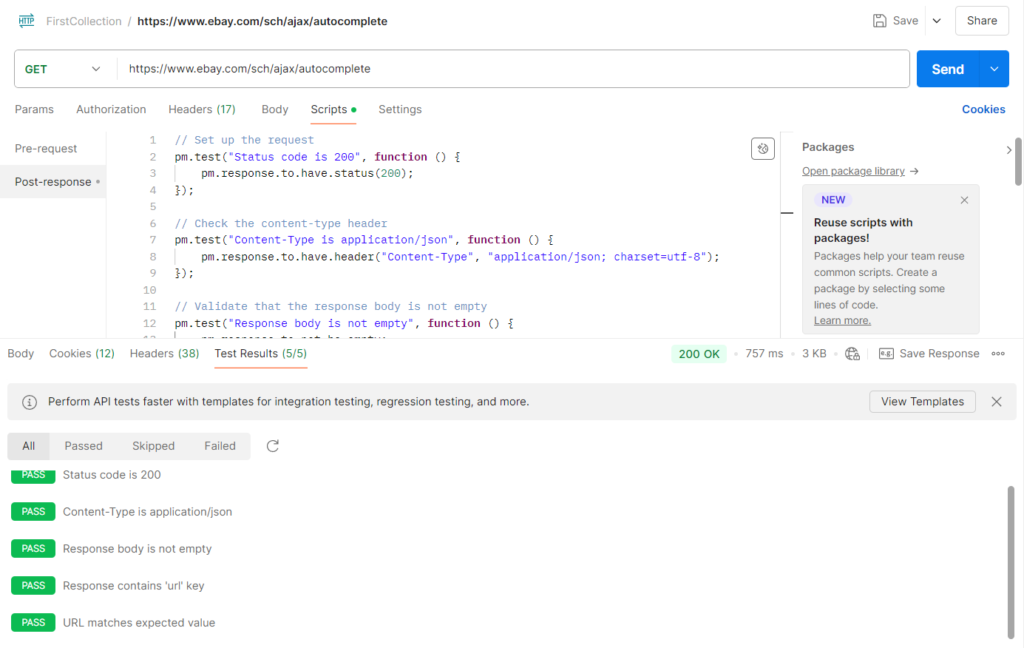
AB_SWITCH_ACC_SIGNOUT
Here is the body text we received by running a GET request for an API token item:
{
"treatment": "C"
}
Here is the list of items tested:
- Status Code is Not 404:
- The test ensures the server doesn’t return a
404 Not Found
, which indicates the resource couldn’t be located.
- Status Code is Valid:
- This checks that the response status is either
200
,201
, or204
, which are common success codes.
- Response Time:
- Ensures that the response time is less than 500ms, which is useful for performance testing.
- Content-Type Validation:
- Checks if the response header includes
Content-Type: application/json
, ensuring that the API returns JSON as expected.
- Response Body Contains ‘treatment’:
- Verifies that the response body has the
treatment
field.
- ‘treatment’ Value is ‘C’:
- Check if the value of the
treatment
field is"C"
to confirm that the API returned the correct data.
And finally, here is the script that runs these requests:
// 1. Test if the status code is not 404
pm.test("Status code is not 404", function () {
pm.expect(pm.response.code).not.to.equal(404);
});
// 2. Test for valid status codes (200, 201, 204)
pm.test("Status code is valid (200, 201, or 204)", function () {
pm.expect(pm.response.code).to.be.oneOf([200, 201, 204]);
});
// 3. Test if the response time is under 500ms
pm.test("Response time is less than 500ms", function () {
pm.expect(pm.response.responseTime).to.be.below(500);
});
// 4. Test if Content-Type is application/json
pm.test("Content-Type is application/json", function () {
pm.response.to.have.header("Content-Type", "application/json");
});
// 5. Test if the response body contains the 'treatment' field
var jsonData = pm.response.json();
pm.test("Response contains 'treatment'", function () {
pm.expect(jsonData).to.have.property("treatment");
});
// 6. Test if the value of 'treatment' is 'C'
pm.test("'treatment' is C", function () {
pm.expect(jsonData.treatment).to.equal("C");
});
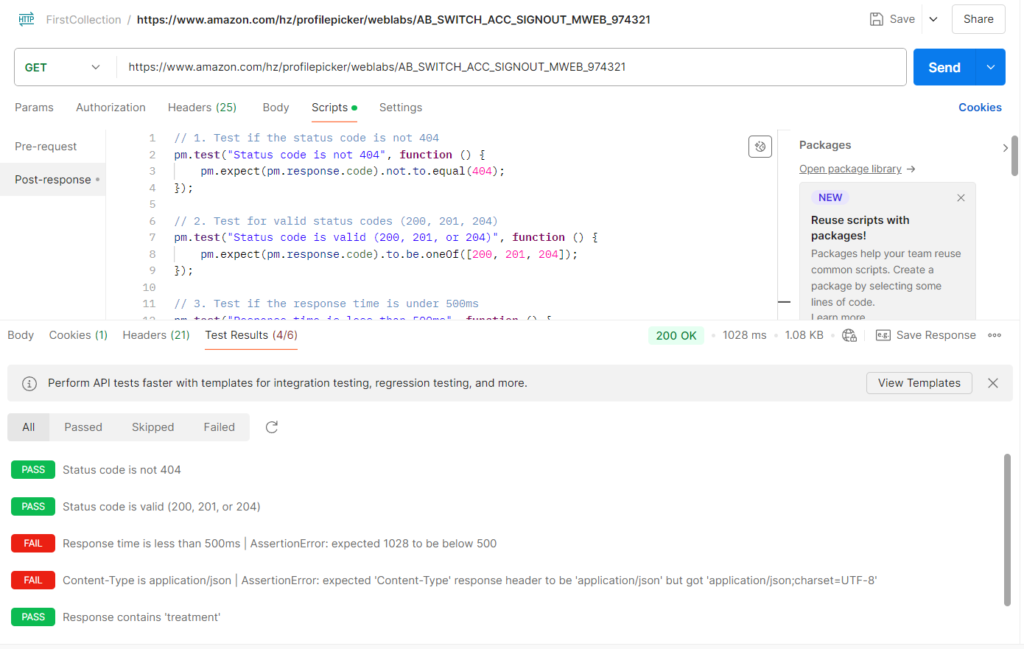
Regarding the Content-Type issue:
The issue you encountered happens because the server is returning the Content-Type
as application/json;charset=UTF-8
, which is valid but differs slightly from what the test is expecting (application/json
). To account for variations like this, we can modify the test to check if the Content-Type
includes "application/json"
instead of exactly matching it.
Here’s an updated version of the test to handle that:
pm.test("Content-Type is application/json", function () {
pm.expect(pm.response.headers.get("Content-Type")).to.include("application/json");
});
Explanation:
- pm.response.headers.get(“Content-Type”): This retrieves the actual
Content-Type
header from the response. - .to.include(“application/json”): This checks whether the
Content-Type
contains"application/json"
, allowing for additional parameters likecharset=UTF-8
to be present without failing the test.
This approach ensures the test passes as long as the response is in JSON format, even if additional information like character encoding is included.
API Token Authentication Test with Response Time Validation
Here is the body text we received by running a POST request for an API token item:
{
"access_token": "_jm579eDPIynflW9PRx7KCL3mZk",
"token_type": "bearer",
"expires_in": 1745,
"scope": "basic openid"
}
Here is the list of items tested:
- Response Time: Verifies that the API response time is less than 200ms.
- HTTP Status Code: Ensures the response status code is
200 OK
. - Response Structure: Checks that the following fields are present in the response:
access_token
token_type
expires_in
scope
- Response Time Logging: Logs the response time for debugging or monitoring purposes.
Here is the Postman script:
pm.test("Response time is less than 200ms", function () {
pm.expect(pm.response.responseTime).to.be.below(200);
});
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
// Check if the correct fields are present in the response
pm.test("Response has all required fields", function () {
const jsonData = pm.response.json();
pm.expect(jsonData).to.have.property("access_token");
pm.expect(jsonData).to.have.property("token_type");
pm.expect(jsonData).to.have.property("expires_in");
pm.expect(jsonData).to.have.property("scope");
});
// Log response time
console.log("Response time: " + pm.response.responseTime + "ms");
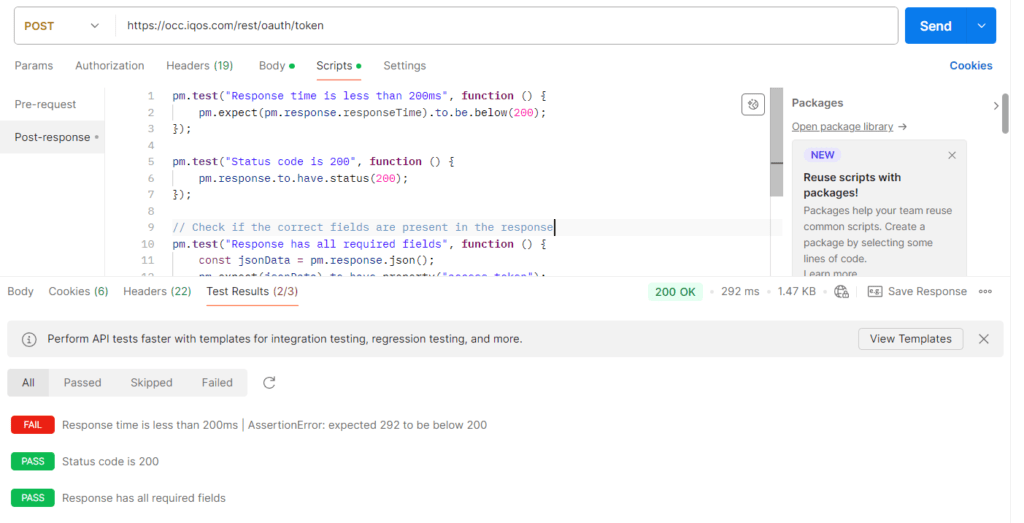
API Response Validation for Chatbot Chat Configuration Data
Here is the body text we received by running a POST request:
{
"key": "GB.en",
"accountSid": "AC828ae166b530b88021314f6009b0764a",
"serviceSid": "IS30842f3a76af3fc0649897f4a2e46719",
"mapSid": "MPe4d0560134514262a40900ff1c98dd2b",
"url": "https://sync.twilio.com/v1/Services/IS30842f3a76af3fc0649897f4a2e46719/Maps/MPe4d0560134514262a40900ff1c98dd2b/Items/GB.en",
"revision": "13",
"data": {
"ChatInputUserNotMemberDisabledText": "You are not a participant of this chat",
"ConfirmEndEngagementConfirmButton": "Yes, I'm done",
"ConfirmEndEngagementDenyButton": "No, take me back",
"ConfirmEndEngagementMessage": "Are you sure you want to end this chat?",
"Connecting": "Connecting …",
"Disconnected": "Connection lost",
"EntryPointTagline": "Start your chat now",
"InputPlaceHolder": "Please write here",
"MessageCanvasTrayButton": "START NEW CHAT",
"MessageCanvasTrayContent": "<h6>Thank you,</h6><p> for contacting IQOS Customer Care.</p> ",
"MessageCharacterCountReached": "Character count reached - {{currentCharCount}} / {{maxCharCount}}",
"MessageInputDisabledReason": "Please select one of the options",
"MessageSendingDisabled": "You are no longer able to send messages",
"NotificationMessage": "This action could not be completed due to a technical error: {{message}}",
"PendingEngagementCancelButton": "Cancel",
"PendingEngagementMessage": "One moment please. My colleague from IQOS CARE will be there for you shortly.",
"PreEngagementFooterLabel": "We kindly ask you not to share sensitive, personal or health-related data with the chatbot. If you would like to know more about how we use information about you,please consult our Privacy Policy here https://pmiprivacy.com/en/consumer",
"PreEngagementSubmitLabel": "Start Chat",
"PreEngagementTextBody": "I am always happy to help! I can support with your account, order, products, services or any other topics. To continue, click start chat on the below.",
"PreEngagementWelcomeMessage": "Hello%USER_NAME%, I'm Carey, your digital assistant from IQOS Customer Care.",
"PredefinedChatMessageAuthorName": "IQOS Support",
"PredefinedChatMessageBody": "Hello, welcome to iQOS support!! By continuing with this chat you accept our privacy policy and terms and conditions listed here on iqos.com. Now, how we may help you?",
"Read": "Read",
"Reset": "RESET",
"Save": "SAVE",
"SendMessageTooltip": "Send Message",
"Today": "TODAY",
"TypingIndicator": "{{name}} is typing ... ",
"WelcomeMessage": "Welcome to IQOS Customer Care",
"Yesterday": "YESTERDAY",
"author": "IQOS CARE",
"chatbotName": "CAREY",
"qualtricsSurveyButtonLabel": "",
"qualtricsSurveyLabel": "For more detailed feedback, please use the link below",
"timezone": "Europe/London",
"welcomeMessage": "Welcome to IQOS Customer Care"
},
"dateExpires": null,
"dateCreated": "2024-01-29T13:48:59.000Z",
"dateUpdated": "2024-01-29T13:48:59.000Z",
"createdBy": "system"
}
Here is the list of tested items as well as the script:
- The script checks that the status code is 200.
- It verifies that the response contains certain fields like
key
,accountSid
,serviceSid
,mapSid
, andurl
, with the expected values. - The script further validates the
data
object, ensuring it has key fields likeChatInputUserNotMemberDisabledText
,ConfirmEndEngagementConfirmButton
, andPreEngagementWelcomeMessage
. - The script ensures
dateCreated
anddateUpdated
are not null, and that thecreatedBy
field exists and is equal to"system"
.
// Parse the response body
let responseData = pm.response.json();
// Status code check
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
// Verify that key fields exist
pm.test("Response contains 'key'", function () {
pm.expect(responseData).to.have.property('key');
pm.expect(responseData.key).to.eql("GB.en");
});
pm.test("Response contains 'accountSid'", function () {
pm.expect(responseData).to.have.property('accountSid');
pm.expect(responseData.accountSid).to.eql("AC828ae166b530b88021314f6009b0764a");
});
pm.test("Response contains 'serviceSid'", function () {
pm.expect(responseData).to.have.property('serviceSid');
pm.expect(responseData.serviceSid).to.eql("IS30842f3a76af3fc0649897f4a2e46719");
});
pm.test("Response contains 'mapSid'", function () {
pm.expect(responseData).to.have.property('mapSid');
pm.expect(responseData.mapSid).to.eql("MPe4d0560134514262a40900ff1c98dd2b");
});
// Verify that 'url' contains the expected structure
pm.test("URL format is correct", function () {
pm.expect(responseData.url).to.eql("https://sync.twilio.com/v1/Services/IS30842f3a76af3fc0649897f4a2e46719/Maps/MPe4d0560134514262a40900ff1c98dd2b/Items/GB.en");
});
// Verify the nested 'data' object exists and contains specific fields
pm.test("'data' object contains expected fields", function () {
pm.expect(responseData.data).to.be.an('object');
pm.expect(responseData.data).to.have.property('ChatInputUserNotMemberDisabledText');
pm.expect(responseData.data.ChatInputUserNotMemberDisabledText).to.eql("You are not a participant of this chat");
pm.expect(responseData.data).to.have.property('ConfirmEndEngagementConfirmButton');
pm.expect(responseData.data.ConfirmEndEngagementConfirmButton).to.eql("Yes, I'm done");
pm.expect(responseData.data).to.have.property('PreEngagementWelcomeMessage');
pm.expect(responseData.data.PreEngagementWelcomeMessage).to.eql("Hello%USER_NAME%, I'm Carey, your digital assistant from IQOS Customer Care.");
});
// Verify non-null 'dateCreated' and 'dateUpdated'
pm.test("'dateCreated' and 'dateUpdated' are valid", function () {
pm.expect(responseData.dateCreated).to.not.be.null;
pm.expect(responseData.dateUpdated).to.not.be.null;
});
// Additional test for 'createdBy'
pm.test("'createdBy' field exists and equals 'system'", function () {
pm.expect(responseData).to.have.property('createdBy');
pm.expect(responseData.createdBy).to.eql('system');
});
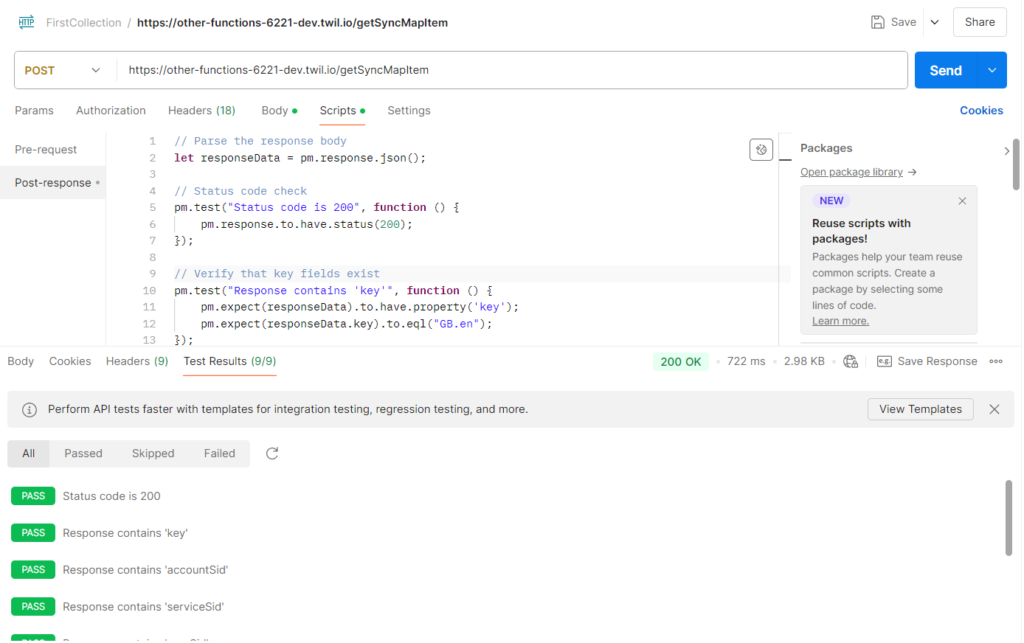
The test’s purpose: validating the API response from Twilio Sync, specifically focusing on chat configuration data such as user messages, buttons, and engagement texts, as well as key metadata like service IDs and creation dates.
Product price and currency validation
Here is the body text we received by running a POST request:
{
"currentPage": 0,
"products": [
{
"ageVerified": false,
"code": "TEREAMixedBundle",
"groupedByBaseProduct": false,
"isRedemptionEligible": false,
"isRewardEligible": false,
"name": "TEREA Mixed Bundle",
"pack": false,
"preOrderable": false,
"price": {
"currencyIso": "GBP",
"formattedValue": "£70.00",
"priceType": "BUY",
"value": 70.0
},
"seoNoArchive": false,
"seoNoFollow": false,
"seoNoIndex": false,
"stock": {
"stockLevel": 0,
"stockLevelStatus": "outOfStock"
},
"subjectedToRegistration": false,
"variantProduct": false
},
{
"ageVerified": false,
"code": "PEARLSAboraOuterBundle",
"groupedByBaseProduct": false,
"isRedemptionEligible": false,
"isRewardEligible": false,
"name": "PEARLS Abora & Outer Bundle",
"pack": false,
"preOrderable": false,
"price": {
"currencyIso": "GBP",
"formattedValue": "£77.00",
"priceType": "BUY",
"value": 77.0
},
"seoNoArchive": false,
"seoNoFollow": false,
"seoNoIndex": false,
"stock": {
"stockLevel": 0,
"stockLevelStatus": "outOfStock"
},
"subjectedToRegistration": false,
"variantProduct": false
},
{
"ageVerified": false,
"code": "G0000717",
"groupedByBaseProduct": false,
"isRedemptionEligible": false,
"isRewardEligible": false,
"name": "TEREA Amber (Classic Tobacco)",
"pack": false,
"preOrderable": false,
"price": {
"currencyIso": "GBP",
"formattedValue": "£70.00",
"priceType": "BUY",
"value": 70.0
},
"seoNoArchive": false,
"seoNoFollow": false,
"seoNoIndex": false,
"stock": {
"stockLevel": 2372,
"stockLevelStatus": "inStock"
},
"subjectedToRegistration": false,
"variantProduct": false
},
{
"ageVerified": false,
"code": "G0000724",
"groupedByBaseProduct": false,
"isRedemptionEligible": false,
"isRewardEligible": false,
"name": "TEREA Green (Menthol)",
"pack": false,
"preOrderable": false,
"price": {
"currencyIso": "GBP",
"formattedValue": "£70.00",
"priceType": "BUY",
"value": 70.0
},
"seoNoArchive": false,
"seoNoFollow": false,
"seoNoIndex": false,
"stock": {
"stockLevel": 1765,
"stockLevelStatus": "inStock"
},
"subjectedToRegistration": false,
"variantProduct": false
},
{
"ageVerified": false,
"code": "G0000738",
"groupedByBaseProduct": false,
"isRedemptionEligible": false,
"isRewardEligible": false,
"name": "TEREA TURQUOISE BUNDLE (10)",
"pack": false,
"preOrderable": false,
"price": {
"currencyIso": "GBP",
"formattedValue": "£70.00",
"priceType": "BUY",
"value": 70.0
},
"seoNoArchive": false,
"seoNoFollow": false,
"seoNoIndex": false,
"stock": {
"stockLevel": 2412,
"stockLevelStatus": "inStock"
},
"subjectedToRegistration": false,
"variantProduct": false
},
{
"ageVerified": false,
"code": "G0000735",
"groupedByBaseProduct": false,
"isRedemptionEligible": false,
"isRewardEligible": false,
"name": "TEREA Teak (Classic Tobacco)",
"pack": false,
"preOrderable": false,
"price": {
"currencyIso": "GBP",
"formattedValue": "£70.00",
"priceType": "BUY",
"value": 70.0
},
"seoNoArchive": false,
"seoNoFollow": false,
"seoNoIndex": false,
"stock": {
"stockLevel": 1686,
"stockLevelStatus": "inStock"
},
"subjectedToRegistration": false,
"variantProduct": false
},
{
"ageVerified": false,
"code": "G0000951",
"groupedByBaseProduct": false,
"isRedemptionEligible": false,
"isRewardEligible": false,
"name": "TEREA ABORA PEARL BUNDLE (Capsule)",
"pack": false,
"preOrderable": false,
"price": {
"currencyIso": "GBP",
"formattedValue": "£70.00",
"priceType": "BUY",
"value": 70.0
},
"seoNoArchive": false,
"seoNoFollow": false,
"seoNoIndex": false,
"stock": {
"stockLevel": 1882,
"stockLevelStatus": "inStock"
},
"subjectedToRegistration": false,
"variantProduct": false
}
],
"totalPageCount": 0,
"totalProductCount": 0
}
Here is the list of tested items as well as the script:
- Extract the product array from the response body.
- Loop through each product and verify the price value and currency.
// Parse the response body
let responseData = pm.response.json();
// Function to check product price
function checkPrice(product, expectedPrice, expectedCurrency) {
pm.test(`Price for product ${product.code} is correct`, function () {
// Check the price value
pm.expect(product.price.value).to.eql(expectedPrice);
// Check the currency
pm.expect(product.price.currencyIso).to.eql(expectedCurrency);
});
}
// Loop through the products
responseData.products.forEach((product) => {
// Define expected price and currency (you can customize this based on your requirements)
let expectedPrice = 70.0;
let expectedCurrency = "GBP";
// Check if product is "PEARLS Abora & Outer Bundle" which has a different price in compare to all other products listed
if (product.code === "PEARLSAboraOuterBundle") {
expectedPrice = 77.0;
}
// Call the function to check the price and currency
checkPrice(product, expectedPrice, expectedCurrency);
});
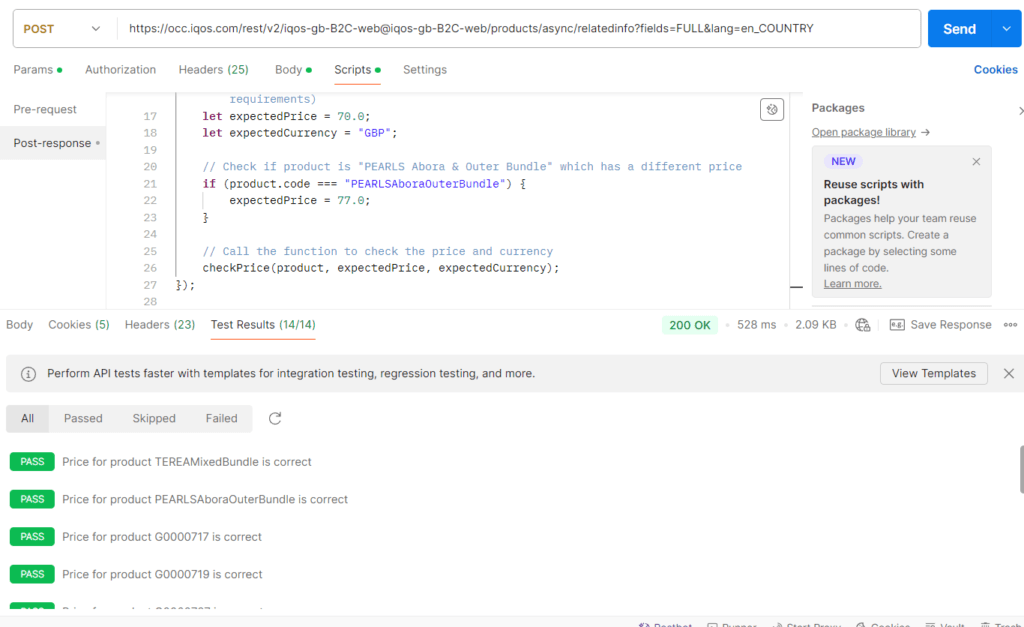