As someone who regularly works with APIs, I know firsthand how essential it is to ensure that everything works smoothly, especially when it comes to managing user authentication and social login flows. Whether you’re handling Single Sign-On (SSO), CAPTCHA systems, or detailed configuration flags, many issues can arise if things aren’t tested properly. That’s where API testing comes in, and one of my favorite tools for the job is Postman.
Recently, I had the opportunity to work with an API response from a system that manages SSO, CAPTCHA settings, and various configuration flags. In this post, I will share how I approached testing such a complex API using Postman and how you can do the same. This kind of testing can help solve several key problems:
- Ensuring Correct Login Behavior: Whether it’s social login using providers like Google or standard username-password authentication, it’s crucial to ensure the login flow is correct and secure.
- Validating Configuration Flags: These flags determine how different system parts behave, such as whether cookies are handled securely or how the interface adapts to accessibility needs (WCAG compliance).
- Testing Domain and SSO Settings: With multiple domains and subdomains, verifying that only authorized domains can access user data or log in via SSO is important.
What I Was Testing
Here’s the body of an API response I received from a GET request:
{
"callId": "c569ee9e5e3346639cf9ed6079aca16a",
"errorCode": 0,
"statusCode": 200,
"statusReason": "OK",
"time": "2024-09-16T20:57:13.728Z",
"flags": {
"addGigSocialLoginParamToRedirectUrl": false,
"alignSocialWidget": true,
"alwaysCheckCookieSave": true,
"checkTokenRenewDeprecation": false,
"fixPhoneTFATranslations": true,
"removeAkamaiEdgeControlHeader": true,
"setCookieSameSiteLaxSession": true,
"verifyLoginOnlyOnce": true
},
"dataCenter": "eu1",
"api": {
"ssoKey": "3_1DHNEexkFbWqmVBs2ycfavVdF5lS6RXiW1b04wrqaMm6c1umCGXTZ8KdUwomQkbg",
"gmidTicketExpiration": 3600,
"baseDomains": [
"iqos.com",
"veev-vape.com"
]
},
"sso": {
"validDomains": [
"gigya.com",
"iqos.com",
"veev-vape.com"
]
},
"captcha": {
"captchaProvider": "Google",
"recaptchaEnterprise": {
"siteKey": "6LdHqBcnAAAAACR0SCiXgGCmcn59uV5UDaEmzXYY"
}
},
"providers": {
"googlePlus": {
"clientId": "801153325598-kjh5er7j26ijaf4cv6gb19rdt2kb5ar8.apps.googleusercontent.com",
"scopes": "profile email"
}
}
}
This response contains several key areas that I needed to test:
- Login and Authentication Settings: I wanted to verify that the login system behaves as expected—especially ensuring that Google OAuth flows work smoothly and that the login only happens once per session.
- Configuration Flags: There are several flags like
alwaysCheckCookieSave
andsetCookieSameSiteLaxSession
that control how cookies are handled. These are vital for secure authentication and compliance with standards like GDPR. - Domain Validation: With multiple valid domains listed, I had to ensure that only those domains could perform SSO. This prevents unauthorized domains from logging in on behalf of users.
- CAPTCHA Integration: Google’s reCAPTCHA system is used for extra security, and I needed to ensure that the correct site key is passed during the login flow.
- OAuth Provider Configurations: Specifically testing the
googlePlus
client ID and ensuring that the scopes (profile
,email
) are correct.
Why Test This?
Testing these settings isn’t just about functionality but security and compliance. If login mechanisms fail, or if cookies are not appropriately handled, users could be exposed to potential security vulnerabilities. Ensuring the correct domains are allowed for SSO prevents malicious actors from redirecting login flows to unauthorized locations. I’m safeguarding the system’s integrity and ensuring a seamless user experience by running these tests.
The Postman Setup
To start testing this API, I created a collection in Postman and divided it into the different areas I wanted to validate.
1. Testing the Authentication Flow + Domain Validation
For authentication, I wrote a test to check if the ssoKey
and baseDomains
are returned correctly, confirming that SSO would work across the expected domains.
pm.test("Check ssoKey is present", function () {
pm.response.to.have.jsonBody("api.ssoKey");
});
pm.test("Valid base domains", function () {
const baseDomains = pm.response.json().api.baseDomains;
pm.expect(baseDomains).to.include("iqos.com");
pm.expect(baseDomains).to.include("veev-vape.com");
});
These tests ensure that the SSO key is being generated and that the correct domains are authorized to use it.
2. Validating Configuration Flags
Next, I tested the configuration flags to ensure they were set to the expected values. This is critical, as these flags control how cookies, login flows, and other security elements are handled.
const flags = pm.response.json().flags;
pm.test("Check flag settings", function () {
pm.expect(flags.alwaysCheckCookieSave).to.be.true;
pm.expect(flags.verifyLoginOnlyOnce).to.be.true;
pm.expect(flags.setCookieSameSiteLaxSession).to.be.true;
});
These tests ensure that important flags like cookie handling and login verification are properly configured.
3. Verifying CAPTCHA Integration
Google’s reCAPTCHA is a vital layer of security, especially for login forms. I wrote a simple test to ensure that the correct site key was being passed for the CAPTCHA.
const captcha = pm.response.json().captcha;
pm.test("Check CAPTCHA provider and siteKey", function () {
pm.expect(captcha.captchaProvider).to.equal("Google");
pm.expect(captcha.recaptchaEnterprise.siteKey).to.equal("6LdHqBcnAAAAACR0SCiXgGCmcn59uV5UDaEmzXYY");
});
This ensures that CAPTCHA is correctly configured and helps prevent spam or bot attacks.
4. Testing OAuth Provider Configurations
Finally, I wanted to make sure the OAuth flow via Google was configured correctly. Specifically, I tested that the client ID and scopes were correct.
const googleProvider = pm.response.json().providers.googlePlus;
pm.test("Google OAuth provider settings", function () {
pm.expect(googleProvider.clientId).to.equal("801153325598-kjh5er7j26ijaf4cv6gb19rdt2kb5ar8.apps.googleusercontent.com");
pm.expect(googleProvider.scopes).to.include("profile");
pm.expect(googleProvider.scopes).to.include("email");
});
This test ensures that the OAuth flow would work properly with Google’s APIs and that the user data being requested is correct.
Putting It All Together
Here’s the final Postman script that ties everything together into a single test collection. It covers testing for SSO, CAPTCHA, configuration flags, and OAuth provider configurations:
// Authentication Tests
pm.test("Check ssoKey is present", function () {
pm.response.to.have.jsonBody("api.ssoKey");
});
pm.test("Valid base domains", function () {
const baseDomains = pm.response.json().api.baseDomains;
pm.expect(baseDomains).to.include("iqos.com");
pm.expect(baseDomains).to.include("veev-vape.com");
});
// Flag Tests
const flags = pm.response.json().flags;
pm.test("Check flag settings", function () {
pm.expect(flags.alwaysCheckCookieSave).to.be.true;
pm.expect(flags.verifyLoginOnlyOnce).to.be.true;
pm.expect(flags.setCookieSameSiteLaxSession).to.be.true;
});
// CAPTCHA Tests
const captcha = pm.response.json().captcha;
pm.test("Check CAPTCHA provider and siteKey", function () {
pm.expect(captcha.captchaProvider).to.equal("Google");
pm.expect(captcha.recaptchaEnterprise.siteKey).to.equal("6LdHqBcnAAAAACR0SCiXgGCmcn59uV5UDaEmzXYY");
});
// OAuth Tests
const googleProvider = pm.response.json().providers.googlePlus;
pm.test("Google OAuth provider settings", function () {
pm.expect(googleProvider.clientId).to.equal("801153325598-kjh5er7j26ijaf4cv6gb19rdt2kb5ar8.apps.googleusercontent.com");
pm.expect(googleProvider.scopes).to.include("profile");
pm.expect(googleProvider.scopes).to.include("email");
});
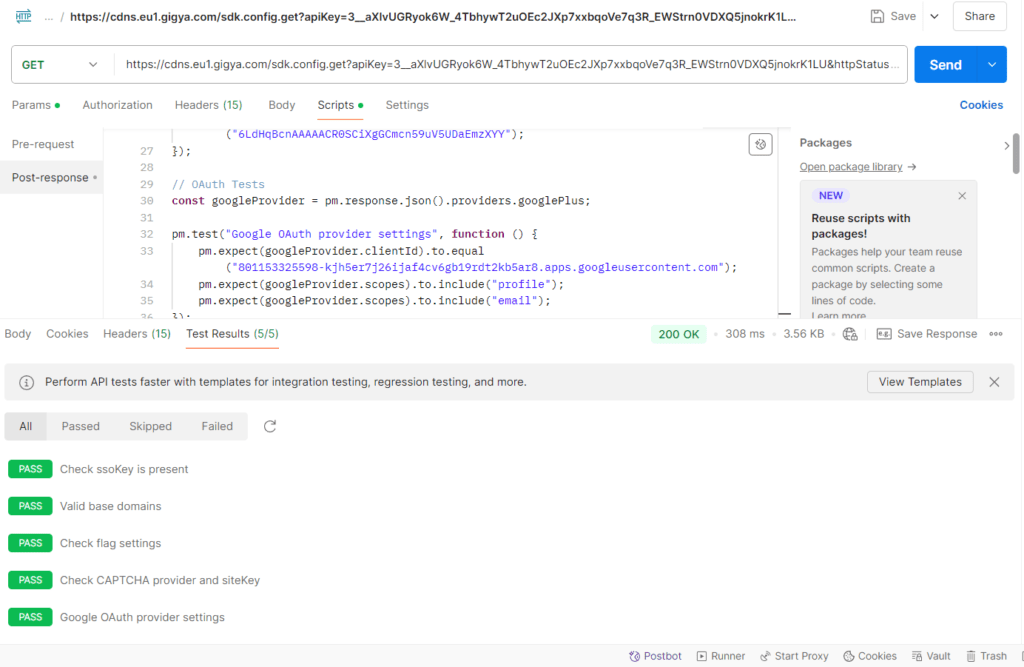