In my previous article on SOAP Salesforce Authorization with Postman (no OAuth), I explained how to set up a Salesforce testing environment with Postman by using the SOAP method for authentication. Basically, the authentication process means sending a login request that will generate a successful response containing the sessionId value we must use in all further tests inside Salesforce. Using it means that we have to set that sessionId into the header of each request after login.
Now, let’s say that we want to automate the process. The login and authentication must be an integral part of this process so it can’t be done separately in terms of generating and implementing the sessionId and then just automating the later steps. It would be impractical.
Let me explain this on a very concrete test case example!
Request 1: Authenticate
Request type: POST
Path: https://login.salesforce.com/services/Soap/u/58.0
Headers:
KEY | VALUE |
Content-Type | text/xml |
SOAPAction | “” |
Body (raw):
<?xml version="1.0" encoding="utf-8" ?>
<env:Envelope xmlns:xsd="http://www.w3.org/2001/XMLSchema"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:env="http://schemas.xmlsoap.org/soap/envelope/">
<env:Body>
<n1:login xmlns:n1="urn:partner.soap.sforce.com">
<n1:username>USERNAME HERE</n1:username>
<n1:password>PASSWORD+TOKEN HERE</n1:password>
</n1:login>
</env:Body>
</env:Envelope>
(Send a request in order to get a response based on which you will have to create the script that will take and print the desired element value)
Response
<?xml version="1.0" encoding="UTF-8"?>
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns="urn:partner.soap.sforce.com" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<soapenv:Body>
<loginResponse>
<result>
<metadataServerUrl>https://fun-inspiration-5108.my.salesforce.com/services/Soap/m/58.0/00DKB000000KQW4</metadataServerUrl>
<passwordExpired>false</passwordExpired>
<sandbox>false</sandbox>
<serverUrl>https://fun-inspiration-5108.my.salesforce.com/services/Soap/u/58.0/00DKB000000KQW4</serverUrl>
<sessionId>00DKB000000KQW4!AR8AQALLEzgl.emG9BJjpziAonaefBtGRgE_hSN8tw01cfN30TgJnK2cgzP8Mefi15fqhl3ZLFm1uS7WXxApTG3R51bqkjdY</sessionId>
<userId>005KB000000JP11YAG</userId>
<userInfo>
<accessibilityMode>false</accessibilityMode>
<chatterExternal>false</chatterExternal>
<currencySymbol>RSD</currencySymbol>
<orgAttachmentFileSizeLimit>5242880</orgAttachmentFileSizeLimit>
<orgDefaultCurrencyIsoCode>RSD</orgDefaultCurrencyIsoCode>
<orgDefaultCurrencyLocale>sr_RS</orgDefaultCurrencyLocale>
<orgDisallowHtmlAttachments>false</orgDisallowHtmlAttachments>
<orgHasPersonAccounts>false</orgHasPersonAccounts>
<organizationId>00DKB000000KQW42AO</organizationId>
<organizationMultiCurrency>false</organizationMultiCurrency>
<organizationName>Petar Skrbic</organizationName>
<profileId>00eKB000001H75cYAC</profileId>
<roleId xsi:nil="true"/>
<sessionSecondsValid>7200</sessionSecondsValid>
<userDefaultCurrencyIsoCode xsi:nil="true"/>
<userEmail>[email protected]</userEmail>
<userFullName>Petar Skrbic</userFullName>
<userId>005KB000000JP11YAG</userId>
<userLanguage>en_US</userLanguage>
<userLocale>sr_RS</userLocale>
<userName>[email protected]</userName>
<userTimeZone>Europe/Belgrade</userTimeZone>
<userType>Standard</userType>
<userUiSkin>Theme3</userUiSkin>
</userInfo>
</result>
</loginResponse>
</soapenv:Body>
</soapenv:Envelope>
We have to store the <sessionId> value from the response as the environment variable. In order to do it we have to update the same request with the following script:
// Parse the SOAP response using Postman's built-in xml2js parser
const xml2js = require('xml2js');
const parser = new xml2js.Parser();
parser.parseString(pm.response.text(), (err, result) => {
if (err) {
console.error('Error parsing XML:', err);
} else {
// Navigate to the sessionId in the parsed XML response
const sessionId = result['soapenv:Envelope']['soapenv:Body'][0]['loginResponse'][0]['result'][0]['sessionId'][0];
// Save the sessionId as an environment variable
pm.environment.set('sessionId', sessionId);
console.log('Session ID:', sessionId);
}
});
If you successfully run the request, the <sessionId> should be inserted into the environment collection and printed in the console.
Request 2: Get records from the Contact Object
After we have made sure that the <sessionId> key and value are stored in the environment, we have to implement the following variable syntax {{sessionId}} into the header authorization value of the subsequent request. It should take the value of the <sessionId> that has been stored during the previous request.
Request type: GET
Path: https://fun-inspiration-5108.my.salesforce.com/services/data/v58.0/sobjects/Contact
Headers:
KEY | VALUE |
Authorization | Bearer {{sessionId}} |
Response
{
"objectDescribe": {
"activateable": false,
"associateEntityType": null,
"associateParentEntity": null,
"createable": true,
"custom": false,
"customSetting": false,
"deepCloneable": false,
"deletable": true,
"deprecatedAndHidden": false,
"feedEnabled": true,
"hasSubtypes": false,
"isInterface": false,
"isSubtype": false,
"keyPrefix": "003",
"label": "Contact",
"labelPlural": "Contacts",
"layoutable": true,
"mergeable": true,
"mruEnabled": true,
"name": "Contact",
"queryable": true,
"replicateable": true,
"retrieveable": true,
"searchable": true,
"triggerable": true,
"undeletable": true,
"updateable": true,
"urls": {
"compactLayouts": "/services/data/v58.0/sobjects/Contact/describe/compactLayouts",
"rowTemplate": "/services/data/v58.0/sobjects/Contact/{ID}",
"approvalLayouts": "/services/data/v58.0/sobjects/Contact/describe/approvalLayouts",
"listviews": "/services/data/v58.0/sobjects/Contact/listviews",
"describe": "/services/data/v58.0/sobjects/Contact/describe",
"quickActions": "/services/data/v58.0/sobjects/Contact/quickActions",
"layouts": "/services/data/v58.0/sobjects/Contact/describe/layouts",
"sobject": "/services/data/v58.0/sobjects/Contact"
}
},
"recentItems": [
{
"attributes": {
"type": "Contact",
"url": "/services/data/v58.0/sobjects/Contact/003KB000004gli6YAA"
},
"Id": "003KB000004gli6YAA",
"Name": "Great, Peter The"
}
]
}
If we look at the response, inside of the “recentItems” object we will find one item listed, that is, one contact profile. Let’s call that one item by using its ID so that we receive more data about it.
Request 3: GET a single item by its ID number
Now we will use the item ID provided in the previous response (003KB000004gli6YAA) make a request to get additional data about that single item.
Request type: GET
Path: https://fun-inspiration-5108.my.salesforce.com/services/data/v58.0/sobjects/Contact/003KB000004gli6YAA
Headers: (same headers as in Request 2)
KEY | VALUE |
Authorization | Bearer {{sessionId}} |
Body: none
Response
{
"attributes": {
"type": "Contact",
"url": "/services/data/v58.0/sobjects/Contact/003KB000004gli6YAA"
},
"Id": "003KB000004gli6YAA",
"IsDeleted": false,
"MasterRecordId": null,
"AccountId": null,
"LastName": "Great",
"FirstName": "Peter",
"Salutation": "Mr.",
"MiddleName": "The",
"Suffix": null,
"Name": "Peter The Great",
"MailingStreet": "Backo Dusanovo Partizanska 33a",
"MailingCity": "Subotica",
"MailingState": null,
"MailingPostalCode": "24220",
"MailingCountry": "RS",
"MailingLatitude": null,
"MailingLongitude": null,
"MailingGeocodeAccuracy": null,
"MailingAddress": {
"city": "Subotica",
"country": "RS",
"geocodeAccuracy": null,
"latitude": null,
"longitude": null,
"postalCode": "24220",
"state": null,
"street": "Backo Dusanovo Partizanska 33a"
},
"Phone": "0631050515",
"Fax": null,
"MobilePhone": null,
"ReportsToId": null,
"Email": "[email protected]",
"Title": "The Big Boss",
"Department": null,
"OwnerId": "005KB000000JP11YAG",
"CreatedDate": "2025-01-11T19:47:48.000+0000",
"CreatedById": "005KB000000JP11YAG",
"LastModifiedDate": "2025-01-11T19:47:48.000+0000",
"LastModifiedById": "005KB000000JP11YAG",
"SystemModstamp": "2025-01-11T19:47:48.000+0000",
"LastActivityDate": null,
"LastCURequestDate": null,
"LastCUUpdateDate": null,
"LastViewedDate": "2025-01-12T16:59:13.000+0000",
"LastReferencedDate": "2025-01-12T16:59:13.000+0000",
"EmailBouncedReason": null,
"EmailBouncedDate": null,
"IsEmailBounced": false,
"PhotoUrl": "/services/images/photo/003KB000004gli6YAA",
"Jigsaw": null,
"JigsawContactId": null
}
Automating the test
I’ve added just a simple status code test script to each request for this test case purpose.
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
After running the test in Postman Runner, I got these results:
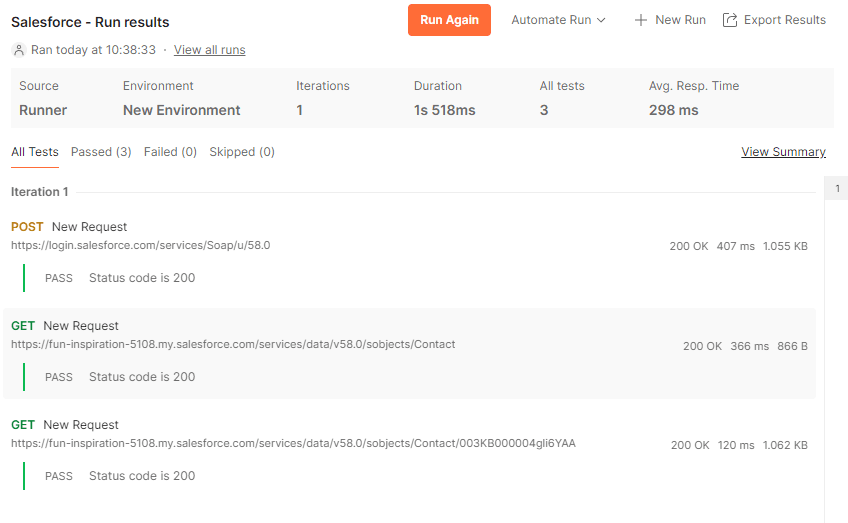
All requests has a Status 200 as expected. In the case that some of the requests have a wrong sessionId value,in that case, it should respond with a status 401 Unauthorized.