I’ve had 2 problematic features on my Area Marker Measur app that is integrated with Google Maps API. By problematic, I meant that they weren’t working correctly. Both of these functions appear inside of the Land box component. First, those are the X buttons for removing the land boxes or the part of the land marked for exclusion. The other is the Show/Hide Side Lengths button, which provides information to the users about the measures of each side of the land unit they marked on the map.
Event Listener 1
The first feature was the X button for removing the Land box or the excluded part of the land.
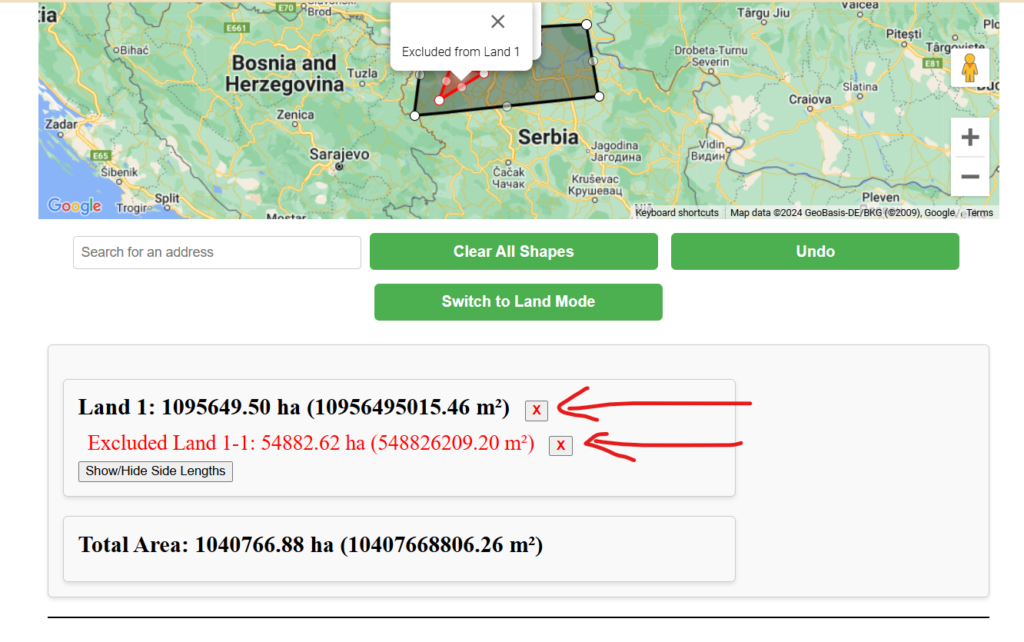
In order to make these work properly, I’ve come up with the following event listener:
// Handle click events for removing land and exclusion overlays
document.getElementById('area').addEventListener('click', function (event) {
const target = event.target;
if (target.tagName === 'BUTTON' && target.dataset.landIndex !== undefined) {
const landIndex = parseInt(target.dataset.landIndex, 10);
const exclusionIndex = parseInt(target.dataset.exclusionIndex, 10);
if (!isNaN(exclusionIndex)) {
console.log(Removing exclusion: landIndex=${landIndex}, exclusionIndex=${exclusionIndex});
// Remove exclusion
removeExclusion(landIndex, exclusionIndex);
} else {
console.log(Removing land: landIndex=${landIndex});
// Remove land
removeLand(landIndex);
}
}
});
It perfectly made the X button work just as I planned.
Event Listener 2
The next solution concerns the functionality of the Show/Hide Side Lengths button, which appears in every land box. By clicking on it, the measures for each side of the marked land unit are shown.
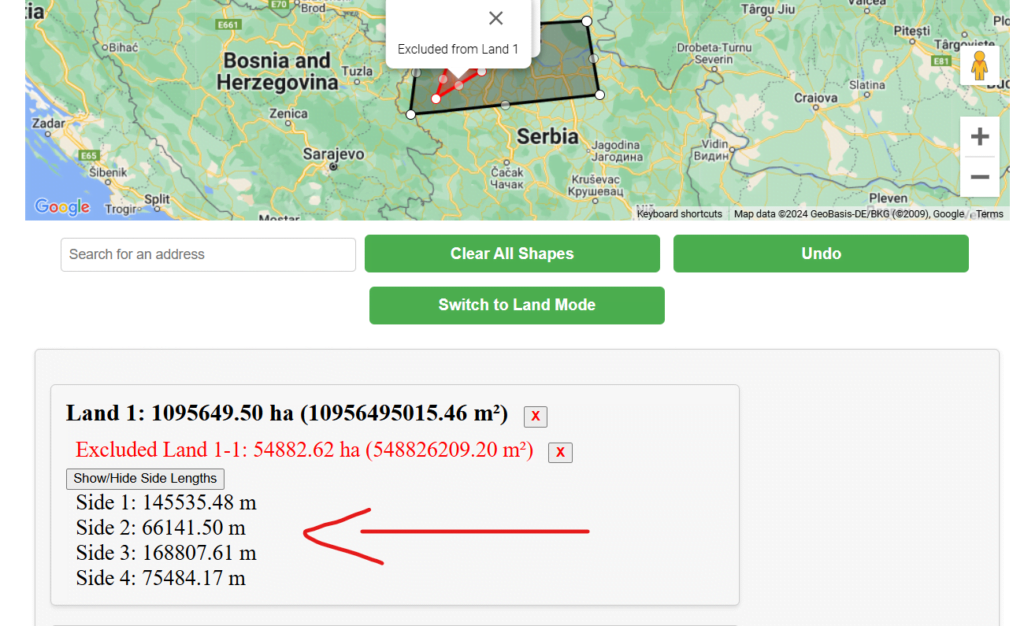
document.getElementById('area').addEventListener('click', function (event) {
const target = event.target;
if (target.tagName === 'BUTTON') {
if (target.classList.contains('delete-land')) {
const landIndex = target.getAttribute('data-land-index');
removeLand(landIndex);
} else if (target.classList.contains('toggle-sides')) {
const sidesIndex = target.getAttribute('data-sides-index');
toggleSideLengths(sidesIndex);
}
}
});
Combined
Now let’s see how it looks when we combine these two Event Listener codes into a single one:
document.getElementById('area').addEventListener('click', function (event) {
const target = event.target;if (target.tagName === 'BUTTON') {
const landIndex = target.getAttribute('data-land-index');
const exclusionIndex = target.getAttribute('data-exclusion-index');
const sidesIndex = target.getAttribute('data-sides-index');
if (landIndex !== null) {
const parsedLandIndex = parseInt(landIndex, 10);
if (!isNaN(exclusionIndex)) {
const parsedExclusionIndex = parseInt(exclusionIndex, 10);
if (!isNaN(parsedExclusionIndex)) {
console.log(`Removing exclusion: landIndex=${parsedLandIndex}, exclusionIndex=${parsedExclusionIndex}`);
removeExclusion(parsedLandIndex, parsedExclusionIndex);
}
} else if (target.classList.contains('delete-land')) {
console.log(`Removing land: landIndex=${parsedLandIndex}`);
removeLand(parsedLandIndex);
}
}
if (sidesIndex !== null && target.classList.contains('toggle-sides')) {
console.log(`Toggling side lengths: sidesIndex=${sidesIndex}`);
toggleSideLengths(sidesIndex);
}
}
});