In this article, we explore a common scenario in web development where user interaction triggers dynamic updates to the UI. Specifically, we’ll look at how to link a button click to an update of a critical component on the page—ensuring that when the user selects a specific area, the application recalculates and displays the updated land size based on the new input.
In this process, we’ll break down the code, starting with the function that calculates and displays the land size. Then, we’ll examine the function that controls the button click, and finally, we’ll connect the two to achieve the desired functionality.
By the end of this article, you’ll understand how to integrate user actions with real-time updates in your web application effectively.
The component that needs to be updated after the button is clicked:
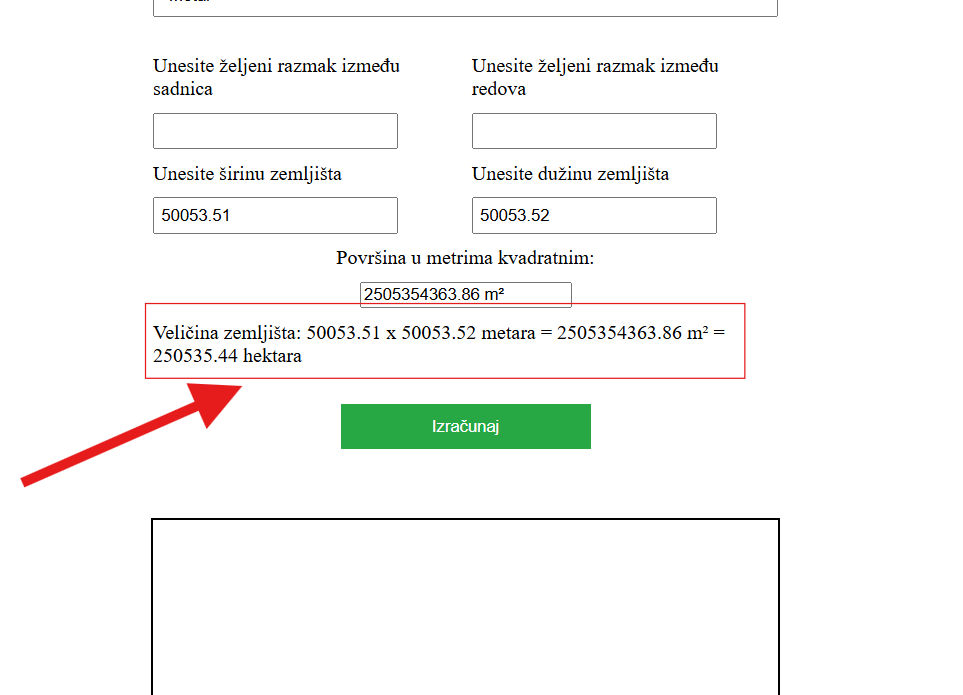
function updateLandSize() {
const landWidth = parseFloat(document.getElementById('landWidth').value);
const landHeight = parseFloat(document.getElementById('landHeight').value);
const unit = document.getElementById('unit').value;
const landSizeDiv = document.getElementById('landSize');
const areaSquareMetersField = document.getElementById('areaSquareMeters');
if (!isNaN(landWidth) && !isNaN(landHeight)) {
let landSizeText = `Veličina zemljišta: ${landWidth} x ${landHeight} ${unit}`;
let area = landWidth * landHeight;
if (unit === 'metara') {
// Update the separate field for square meters
areaSquareMetersField.value = `${area.toFixed(2)} m²`;
landSizeText += ` = ${area.toFixed(2)} m²`;
if (area >= 10000) {
landSizeText += ` = ${(area / 10000).toFixed(2)} hektara`;
}
} else {
// If the unit is not in meters, still show the area in square meters
areaSquareMetersField.value = `${area.toFixed(2)} m²`;
landSizeText += ` = ${area.toFixed(2)} m²`;
}
landSizeDiv.innerHTML = landSizeText;
} else {
// Clear the output fields if the input is invalid
landSizeDiv.innerHTML = '';
areaSquareMetersField.value = '';
}
}
The function that controls the button
In the screenshot, you may see the button that needs to be clicked:
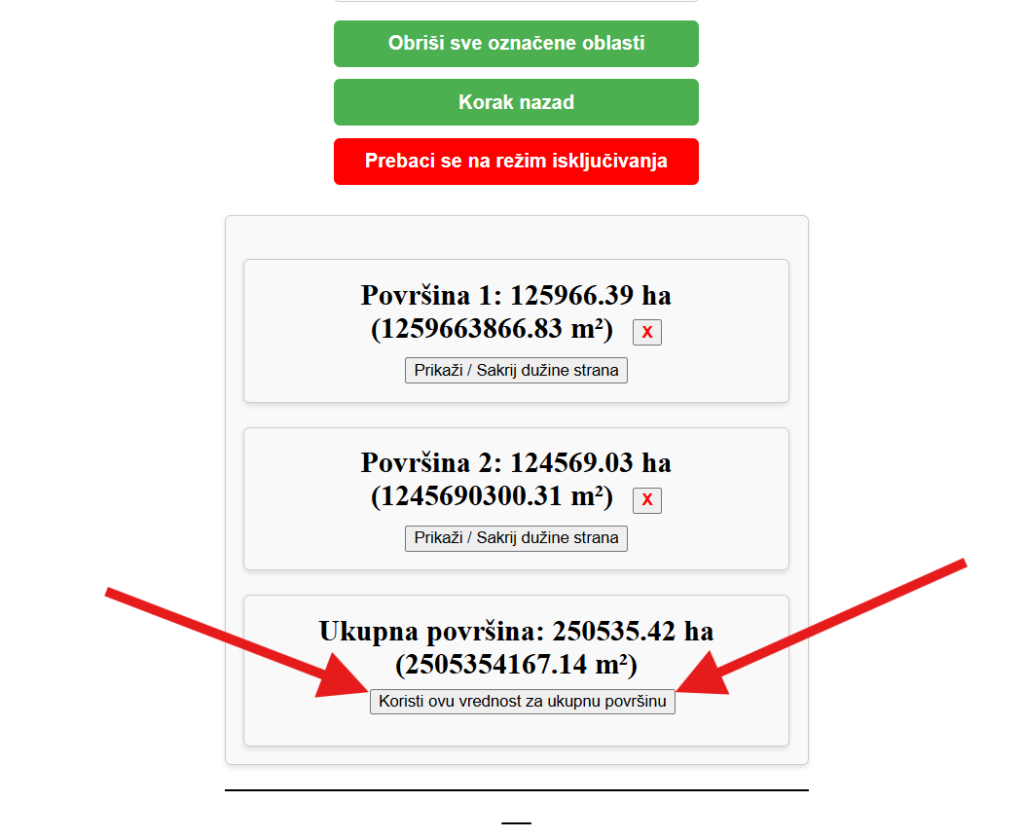
Here is the function related to it:
function updateDisplay() {
let totalArea = 0;
let output = areas.map((areaObj, index) => {
let currentArea = areaObj.area;
let exclusionAreaSum = 0;
const exclusionAreas = areaObj.exclusions.map((exclusionObj, exclusionIndex) => {
const exclusionArea = google.maps.geometry.spherical.computeArea(exclusionObj.overlay.getPath());
exclusionAreaSum += exclusionArea;
return `
<div class="land-details" style="color: red;">
Excluded ${areaObj.label}-${exclusionIndex + 1}: ${(exclusionArea / 10000).toFixed(2)} ha (${exclusionArea.toFixed(2)} m²)
<button data-land-index="${index}" data-exclusion-index="${exclusionIndex}" style="color: red; font-weight: bold; margin-left: 10px;">X</button>
</div>
`;
});
const sideLengths = getSideLengths(areaObj.overlay.getPath());
totalArea += (currentArea - exclusionAreaSum);
return `
<div class="land-box">
<div class="land-header">
${areaObj.label}: ${(currentArea / 10000).toFixed(2)} ha (${currentArea.toFixed(2)} m²)
<button data-land-index="${index}" class="delete-land" style="color: red; font-weight: bold; margin-left: 10px;">X</button>
</div>
${exclusionAreas.join('')}
<button class="toggle-sides" data-sides-index="${index}">Prikaži / Sakrij dužine strana</button>
<div id="sides-${index}" style="display: none;" class="land-details">${sideLengths.map((length, idx) => `<div>Strana ${idx + 1}: ${length.toFixed(2)} m</div>`).join('')}</div>
</div>
`;
}).join('');
// Add the total area display and the new button to copy the value to the input field
output += `
<div class="land-box">
<div class="land-header">
Ukupna površina: ${(totalArea / 10000).toFixed(2)} ha (${totalArea.toFixed(2)} m²)
<button id="copyArea" style="margin-left: 10px;">Koristi ovu vrednost za ukupnu površinu</button>
</div>
</div>`;
document.getElementById('area').innerHTML = output;
The solution:
Basically, the solution lies in connecting these two functions listed above by implementing an event listener into the second function:
document.getElementById('area').addEventListener('click', function (event) {
if (event.target && event.target.id === 'copyArea') {
const totalArea = parseFloat(event.target.closest('.land-header').textContent.match(/([\d,\.]+) m²/)[1].replace(',', ''));
updateLandSize(totalArea);
}
});