As I was working on developing Cypress scripts for testing promotions inside SAP Hybris, I encountered a rather unexpected issue with a seemingly simple component. It was a part of the promotion that displayed a description, and in my mind, I thought, “This component is too basic; nothing can go wrong.” Well, that assumption quickly proved wrong.
The component I was testing contained the description text for a promotion, and my initial approach seemed straightforward enough. I crafted a Cypress command to ensure that the promotion’s description value matched the expected text:
cy.get('.ye-input-text.ye-com_hybris_cockpitng_editor_defaulttext.z-textbox')
.should('have.value', 'All users are able to try a ZYN can as a free sample (or free gift). Limited to 1 per registered user');
Surprisingly, the test failed, showing me an error message that made me scratch my head.
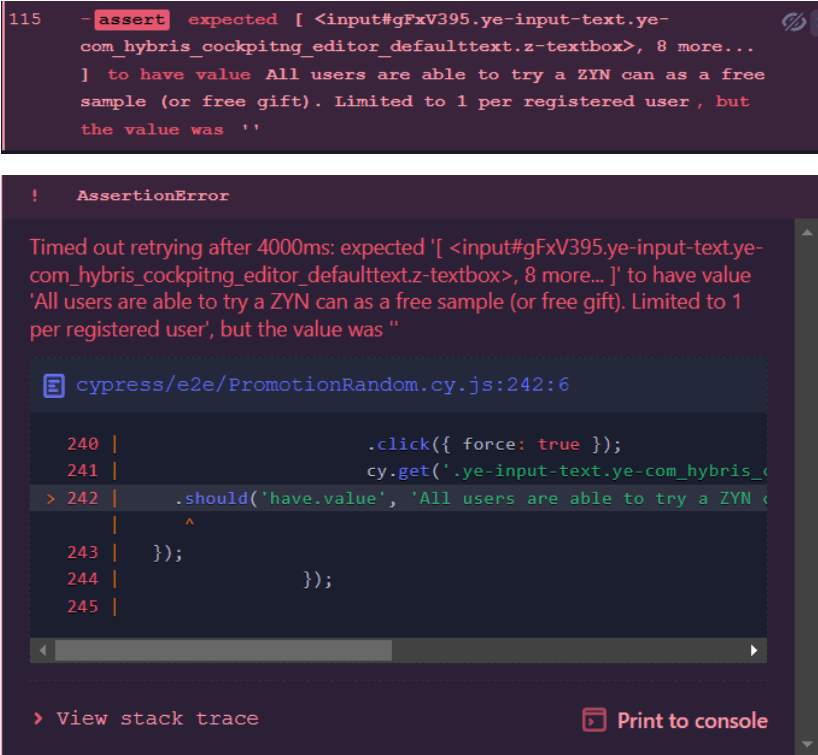
The Cypress log indicated that nine elements were returned by the cy.get()
command because multiple elements shared the same class name. However, none of them contained the value I was expecting. Initially, I thought Cypress would handle this more gracefully—maybe return an error suggesting I use something like .eq()
to pick a specific element. But no such luck; instead, Cypress iterated through all the elements but didn’t find the match I was looking for.
The HTML Structure
The element I was targeting was nested within a series of divs, and this is what the HTML looked like:
<div id="p41H029" class="yw-loceditor-row ye-validation-none z-div">
<span id="p41H129" style="display:none;" class="yw-loceditor-row-locale z-label" title="English">en</span>
<div id="p41H229" class="yw-loceditor-row-editor z-div">
<div id="p41H329" class="z-div">
<input id="p41H429" class="ye-input-text ye-com_hybris_cockpitng_editor_defaulttext z-textbox"
value="All users are able to try a ZYN can as a free sample (or free gift). Limited to 1 per registered user"
type="text" aria-disabled="false" aria-readonly="false">
</div>
</div>
</div>
As you can see, the input field had the correct value, but due to the presence of multiple similar elements, Cypress struggled to pinpoint the exact one.
My Next Attempt
After the initial failure, I decided to refine my approach by narrowing down the scope. I tried using the cy.contains()
command to locate the text and then work my way up to the input field:
cy.contains('All users are able to try a ZYN can as a free sample (or free gift). Limited to 1 per registered user')
.parents('.yw-loceditor-row.z-div') // Go up to the parent row
.find('input') // Then find the input
.should('have.value', 'All users are able to try a ZYN can as a free sample (or free gift). Limited to 1 per registered user');
But, frustratingly, this didn’t work either. The test continued to fail, and I wondered what I was missing.
The Solution: Fine-Tuning the Selector
In essence, when you use .should('have.value', ...)
after a cy.get()
that selects multiple elements, only the first element’s value is tested. This behavior explained why my tests failed: although Cypress found nine elements, none of their values matched because it only checked the first one.
After receiving advice, I refined my selector by using the [value="..."]
attribute to pinpoint the exact element I wanted to test:
cy.get('.ye-input-text.ye-com_hybris_cockpitng_editor_defaulttext.z-textbox[value="${expectedValue}"]')
.should('have.value', '${expectedValue}');
By doing this, I was able to select only the specific input element that contained the promotion description rather than leaving it up to Cypress to search through all the elements with the same class. This method worked perfectly, and the test passed successfully.
Lesson Learned
What seemed like a simple issue was a valuable lesson in precision when writing Cypress scripts. Once I understood how Cypress handles selections, the solution was simple. From now on, I’ll remember to avoid assumptions and be as specific as possible when working with multiple elements that share similar class names.
This experience reminded me that even the smallest components can introduce unexpected challenges, and being thorough in targeting elements can make all the difference in testing accuracy.