Here, I will demonstrate a short Cypress testing procedure for the search bar on a news website. The search bar is an essential feature of news websites in particular. People search for news by keywords, so this feature has to be able to respond appropriately to those inputs. Or, to be more precise, the content matching the input keywords should be served to the users.
Task description
The website I will test here is Informer. It is the leading media house in Serbia in the fields of public manipulation and affair creation. Since Informer’s target audience is primarily searching for the current Serbian president for life, we will test if the website can filter matching news articles on the following keyword: “Vučić.”
Testing steps
Let’s break down the testing process into a few steps:
- Open the following path: https://informer.rs/
- Click on the search icon
- Click on the search bar and type in “Vučić”
- Click on the search (Pretraži) button.
- Filtered articles matching the keyword should appear
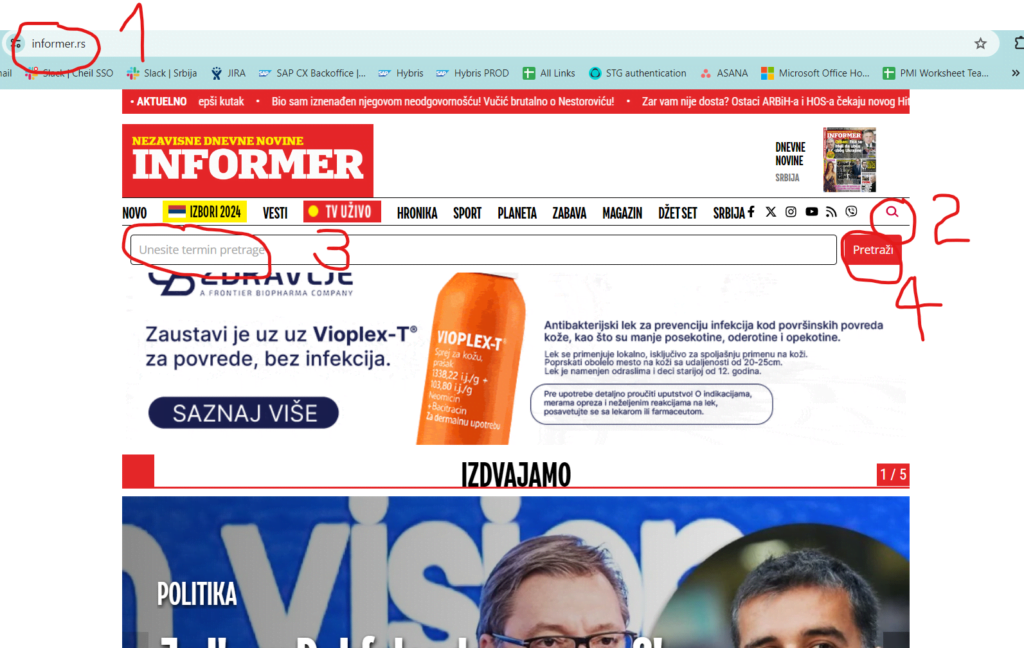
Minor errors I got during the code setup
On every single .click() command, I’ve received the following error:
Timed out retrying after 4050ms:cy.click()
failed because this element is not visible:<span class="toggle-search">...</span>
This element<span.toggle-search>
is not visible because it has CSS property:display: none
Fix this problem, or use{force: true}
to disable error checking.
It was easily solved by implementing {force: true}
into the .click() command: .click({ force: true })
Also, I have easily fixed multiple elements with the same class name by implementing the .eq() command.
The major error was the Googletag is not defined error, which I will cover in the next chapter.
Googletag is not defined Error
After I had successfully managed to set a code that can type in the keyword in the search bar and search for it, I received the following error:
ReferenceError
The following error originated from your application code, not from Cypress.
> googletag is not defined
When Cypress detects uncaught errors originating from your application it will automatically fail the current test. This behavior is configurable, and you can choose to turn this off by listening to the uncaught:exception event.
We can solve this by implementing the following piece of code on the very top of the Cypress code:
Cypress.on('uncaught:exception', (err, runnable) => {
// Return false to prevent the test from failing
return false;
});
This step doesn’t actually “solve” the error at root but ignores it since it isn’t relevant to our testing procedure.
Final code
Here is the Cypress code that properly follows the steps listed above and leading to the expected testing results:
Cypress.on('uncaught:exception', (err, runnable) => {
// Return false to prevent the test from failing
return false;
});
describe('Testing Informers search bar', () => {
it('Click on the search bar, type in "Vučić" and search for it', () => {
// Visit the specified URL
cy.visit('https://informer.rs/');
// Click on the search toggle
cy.get('.toggle-search')
.eq(0)
.click({ force: true });
// Force click on the search input and type 'Vučić'
cy.get('.form-control.search-form-input')
.should('exist')
.click({ force: true })
.type('Vučić', { force: true });
// Click on the search button
cy.get('span.btn-text')
.eq(1)
.click({ force: true });
});
});
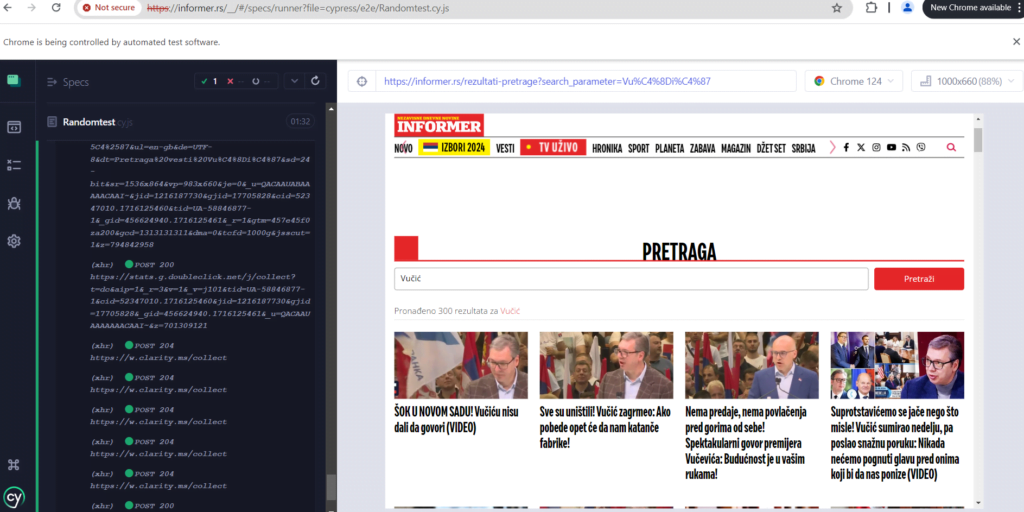