In my journey to optimize Cypress tests for promotions in an online backoffice system, I ran into a specific challenge while testing the “Qualifying Products” section of promotions. This section allows multiple product codes to be associated with a promotion, and I needed to ensure my tests could dynamically handle multiple products efficiently, user-friendly.
Here’s how I solved it by making Cypress tests more flexible and efficient with dynamic product code verification.
The Challenge
When dealing with the “Qualifying Products” section, the test needed to check if the correct products were listed for a given promotion. However, in many cases, multiple products could be associated with a single promotion, and writing separate Cypress commands for each one would be tedious and inefficient.
To streamline the process, I wanted to:
- Allow multiple product codes to be entered into a single Testing Section. So instead of opening 10 Testing Sections for each product code, I wanted to optimize the Testing Section so that the user can insert all product codes at once.
- Dynamically generate Cypress commands that check each product code within the same promotion context.
- Avoid repetitive code by consolidating steps that open and check the necessary components.
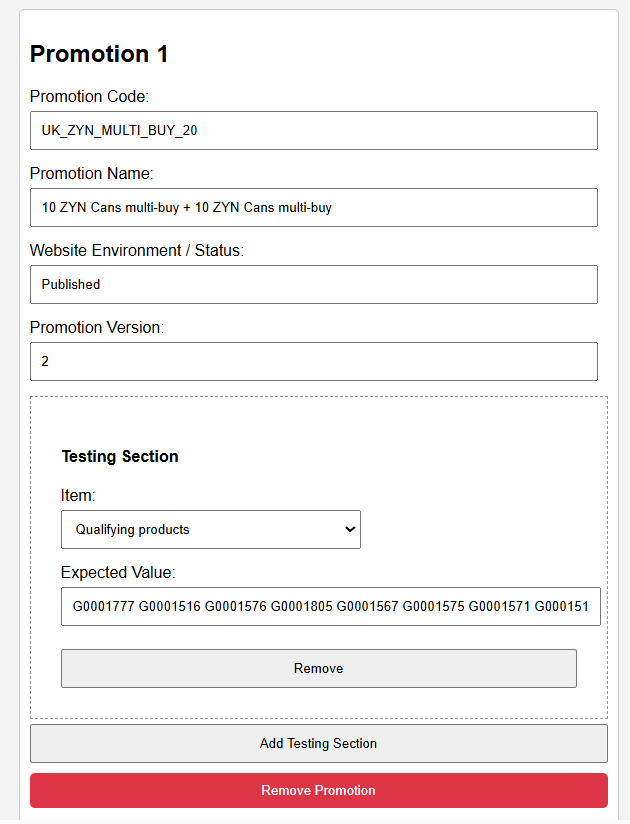
The Solution
I developed a solution where users could input multiple product codes, separated by spaces, and the app would split these values to generate Cypress commands for each product individually. This ensured that the test script was generated dynamically, making it both scalable and efficient.
Here’s the core of the solution:
case 'Qualifying products':
// Split the expected value (product codes) by spaces and generate commands for each product code
const productCodes = expectedValue.split(' '); // Split the product codes by space
productCodes.forEach(productCode => {
commands += `
// Step 1 - Navigate to 'Conditions & Actions' tab
cy.get('.z-tab-content')
.contains('Conditions & Actions')
.scrollIntoView()
.click({ force: true });
// Step 2: Open 'Container' if not already open
cy.get('body').then($body => {
if ($body.find('.z-label:contains("Container")').length > 0) {
cy.get('.z-label')
.contains('Container')
.scrollIntoView()
.then(($container) => {
if (!$container.hasClass('open')) {
cy.wrap($container).click({ force: true });
}
});
} else {
cy.log('Container not found, skipping to the next step.');
}
});
// Step 3: Open 'Qualifying products' if not already open
cy.get('span.z-label').contains('Qualifying products').then(($label) => {
const parent = $label.closest('div.yrb-condition');
cy.wrap(parent).find('i').then(($icon) => {
if ($icon.hasClass('z-icon-caret-right')) {
cy.wrap($label).click();
} else if ($icon.hasClass('z-icon-caret-down')) {
cy.log('Qualifying products is already open');
}
});
});
// Step 4: Verify the product text in the component for each product code
cy.get('span.ye-default-reference-editor-selected-item-label.z-label')
.should('contain.text', '[${productCode}] - ${country} Product Catalog : ${catalogEnv}');
`;
});
break;
Breaking Down the Solution
1. Splitting Product Codes
The first key step was to split the user input (a string of product codes) into individual product codes. This allowed me to loop through each code and generate a Cypress command to test it. The split()
method did the trick here, separating product codes by spaces:
const productCodes = expectedValue.split(' ');
2. Navigating to the ‘Conditions & Actions’ Tab
The Cypress command starts by navigating to the “Conditions & Actions” tab. This step is important as the “Qualifying Products” section is nested within this tab. I ensured the tab was scrolled into view and clicked even if it was outside the viewport:
cy.get('.z-tab-content')
.contains('Conditions & Actions')
.scrollIntoView()
.click({ force: true });
3. Opening the ‘Container’ Section
The next challenge was to check if the “Container” section was open, as the “Qualifying Products” section is often inside it. If it wasn’t open, the script would click to expand it. This was done using conditional logic to avoid unnecessary clicks:
cy.get('body').then($body => {
if ($body.find('.z-label:contains("Container")').length > 0) {
cy.get('.z-label')
.contains('Container')
.scrollIntoView()
.then(($container) => {
if (!$container.hasClass('open')) {
cy.wrap($container).click({ force: true });
}
});
}
});
4. Handling the ‘Qualifying Products’ Section
Similarly, I handled the “Qualifying Products” section with conditional logic. If the section wasn’t open, the script would click the caret to expand it; otherwise, it would log that the section was already open:
cy.get('span.z-label').contains('Qualifying products').then(($label) => {
const parent = $label.closest('div.yrb-condition');
cy.wrap(parent).find('i').then(($icon) => {
if ($icon.hasClass('z-icon-caret-right')) {
cy.wrap($label).click();
} else if ($icon.hasClass('z-icon-caret-down')) {
cy.log('Qualifying products is already open');
}
});
});
5. Verifying Each Product Code
Finally, the test would verify that each product code entered by the user was correctly displayed. This was done dynamically by looping through the list of product codes and checking if each code appeared in the UI:
cy.get('span.ye-default-reference-editor-selected-item-label.z-label')
.should('contain.text', '[${productCode}] - ${country} Product Catalog : ${catalogEnv}');
The Result
This approach significantly improved the efficiency and flexibility of the Cypress tests. Users could now input multiple product codes, and the app would automatically generate the necessary Cypress commands to verify each code. This not only made the script generation process smoother but also reduced manual effort by handling multiple products within a single test case.
By dynamically managing navigation, section toggling, and verification steps, I was able to create a more user-friendly and scalable Cypress testing experience. Now, testing promotions with multiple qualifying products is seamless and efficient, all within a single test case!