Here, I am presenting to you an application that prints the data from your Salesforce object in JSON format in the Visual Studio Code terminal. It easily manages to log into your account by using username, password, and token credentials. No OAuth is used.
You may find the app repository here:
https://github.com/NoToolsNoCraft/GET-Salesforce-Object-Record-in-VSC-Terminal
Set up the app on your device
In order to set up this app on your device, first you have to download it as you wish. The easiest way is by using the Download as ZIP method in GitHub. Unzip the file after downloaded and update it to Visual Studio Code.
Everything is pretty much set up in files; you will only have to apply a few things:
Install dependencies that are listed in the package.json file by running this command:
npm install
Add your Salesforce credentials (username, password & token) to the controller.salesforce.js file
lib > controller > controller.salesforce.js
Also, in the same file, you have the ability to set the object from which you want to take data.
As soon as you are done with the steps above, the next thing you have to do is run the following command in your root directory:
node index.js
This will run the server on the localhost:9005, as it is currently set, although you can change the port to any that fits you. Open the port in the browser, it won’t load any content on the page, it will just keep loading. As soon as you have loaded the localhost in your browser, the Salesforce data will get loaded in your Visual Studio Code terminal as presented in the image on the right (or below if you are reading this from a mobile device).
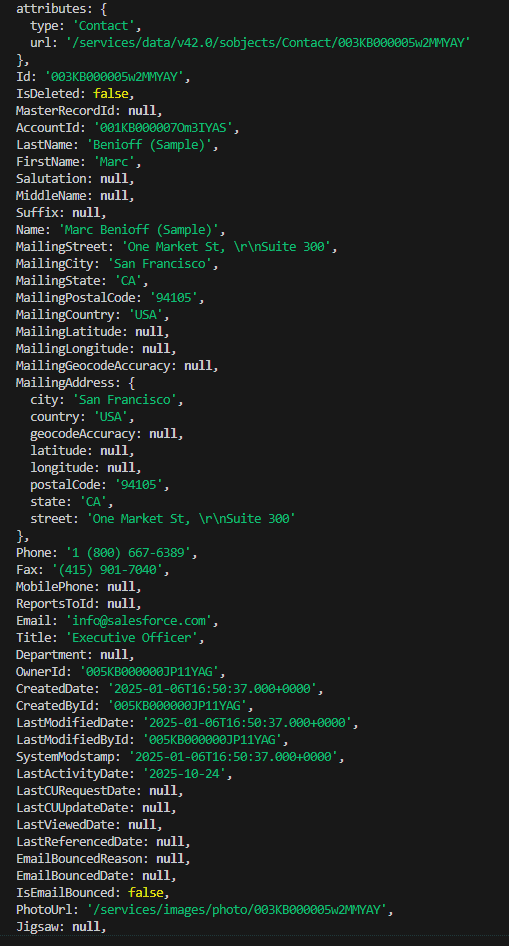
Enable API Access in Salesforce Profile
Before you try to run the app or if you encounter some issues, make sure to check if the API Access is enabled in your Salesforce account. You may find a more detailed explanation of how to do that here: https://notoolsnocraft.tech/enabling-api-access-in-salesforce-profiles/
Update credentials
Navigate to the following file: lib > controller > controller.salesforce.js
Inside the code, you will find this part where you have to update your Salesforce credentials:
var SALESFORCE_USERNAME = "YOUR SALESFORCE USERNAME HERE";
var SALESFORCE_PASSWORD = "YOUR SALESFORCE PASSWORD HERE";
var SALESFORCE_TOKEN = "YOUR SALESFORCE TOKEN HERE";
Update environment
Navigate to the following file: lib > controller > controller.salesforce.js
Inside the code, you will find this part where you have to update your Salesforce environment:
//var SALESFORCE_LOGIN_URL = "https://test.salesforce.com"; // for sandbox
var SALESFORCE_LOGIN_URL = "https://login.salesforce.com"; // for production
Choose the object
Navigate to the following file: lib > controller > controller.salesforce.js
Inside the code, you will find this part where you have to update your Salesforce object which you want to test:
if (loginRes) {
//loginRes.sobject('Opportunity').find({}).execute(function (err, records) {
//loginRes.sobject('Account').find({}).execute(function (err, records) {
//loginRes.sobject('Task').find({}).execute(function (err, records) {
loginRes.sobject('Contact').find({}).execute(function (err, records) {
Currently the Contact object is active, while there are some other objects commented out. Make sure to have only one object active.