Yesterday, Gleb Bahmutov demonstrated a more effective scroll behavior method in Cypress in his new YouTube video. As an avid follower of his work for some time, I have found his insights invaluable for improving my testing processes. Gleb’s ability to break down complex concepts into manageable and practical applications has consistently enhanced my understanding and application of Cypress.
Naturally, I am eager to test his methodologies, often leading to more efficient and reliable testing outcomes. His latest demonstration on scroll behavior is no exception, and I am excited to delve into it and see firsthand how it can optimize my workflows.
Scroll behavior
Cypress, by default, scrolls the input to the top before typing, which can sometimes lead to suboptimal test results or unexpected behaviors, especially when dealing with dynamically loaded content or elements positioned off-screen.
To address this, Gleb introduced a scroll behavior setting that allows testers to control how Cypress scrolls elements into view during interactions. This setting is instrumental in ensuring that elements are centered in the viewport, reducing the chances of missed clicks or hidden elements that can cause test failures.
Implementing this scroll behavior improves the accuracy of automated tests and enhances their reliability by ensuring a consistent view of the test environment. I will discuss the implementation and benefits of this setting, drawing from Gleb’s demonstration and my own testing experiences.
Let’s demonstrate it
I will demonstrate this scrolling behavior on the very simple example of the Vimeo search bar that appears in the Google search results:
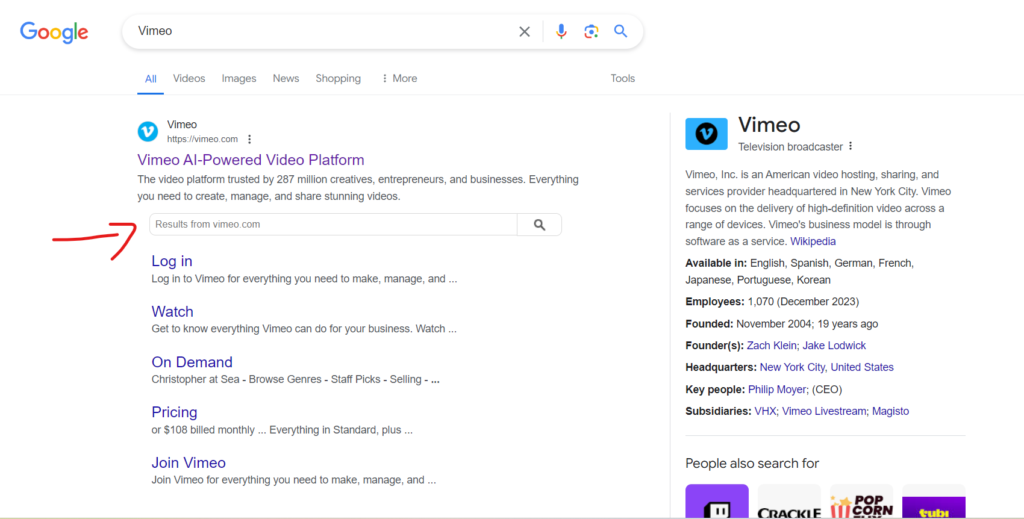
To reach this Vimeo search bar with Cypress, we will need only a few simple steps:
- Search for the “Vimeo” query in Google search: https://www.google.com/search?q=Vimeo
- Locate the Vimeo search bar on the Google search results page.
- Type in the Vimeo search bar (“terminator” in our example)
Here is a piece of code that follows the steps listed above:
describe('Vimeo Google Search results search bar test', () => {
it('should open Google and type Vimeo in the search bar',
() => {
// Open Google search
cy.viewport(1000, 1000)
cy.visit('https://www.google.com/search?q=Vimeo');
//Since the Vimeo search component doesn't always appear after directly reaching the link above we have to click on the Google search button in order to get the resuts we are looking for.
cy.get('div.zgAlFc').click()
//Type in the Vimeo.com search bar
cy.get('input[placeholder="Results from vimeo.com"]').should('be.visible')
cy.get('input[placeholder="Results from vimeo.com"]').type('terminator');
});
});
After running this code, we successfully reached the Vimeo search bar as initially planned. Still, as we can see in the screenshot below when Cypress is typing in the input field, it scrolls the field to the top by default.
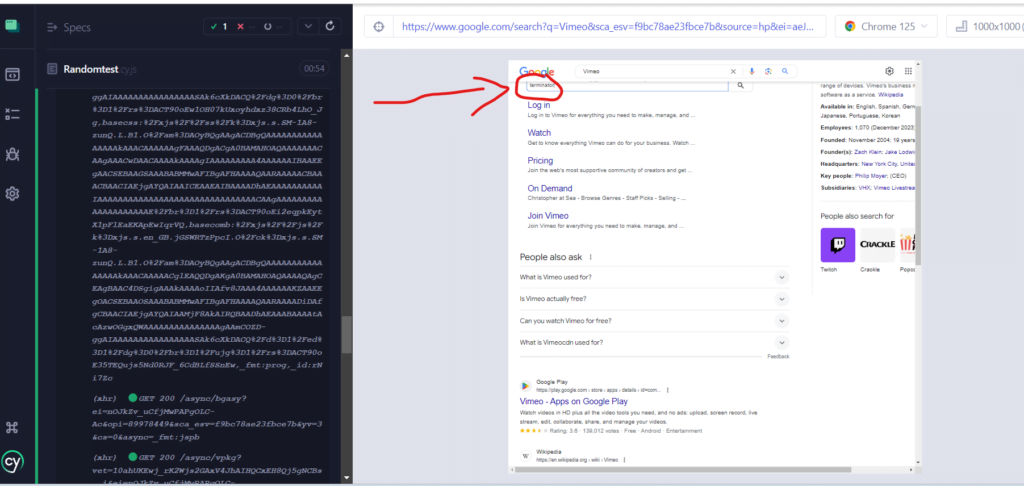
To manage this, we have to implement the following setting into our code:
{
scrollBehavior: 'center',
},
This setting will basically try to scroll the input field to the center of the viewport. In our case, it will keep the viewport as it is without scrolling the input field to the top while typing in it.
Final code:
We have to implement the command above in the code just as it is done here:
describe('Vimeo Google Search results search bar test', () => {
it('should open Google and type Vimeo in the search bar',
{
scrollBehavior: 'center',
},
() => {
// Open Google search
cy.viewport(1000, 1000)
cy.visit('https://www.google.com/search?q=Vimeo');
//Since the Vimeo search component doesn't always appear after directly reaching the link above we have to click on the Google search button in order to get the resuts we are looking for.
cy.get('div.zgAlFc').click()
//Type in the Vimeo.com search bar
cy.get('input[placeholder="Results from vimeo.com"]').should('be.visible')
cy.get('input[placeholder="Results from vimeo.com"]').type('terminator');
});
});
This time, Cypress managed to type “terminator” in the Vimeo search bar without scrolling the field to the top.
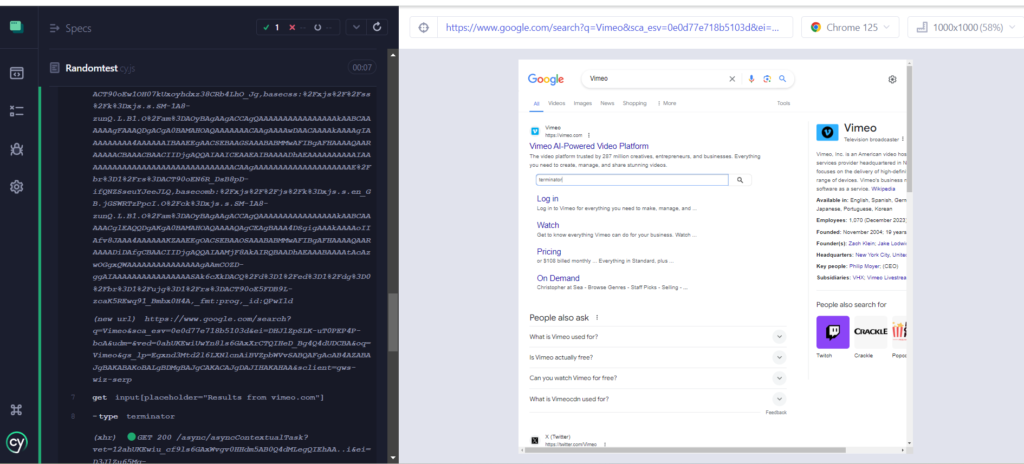