Query the list of # starting with vowels
Query the list of CITY names beginning with vowels (i.e., a
, e
, i
, o
, or u
) from STATION. Your result cannot contain duplicates.
The STATION table is described as follows:
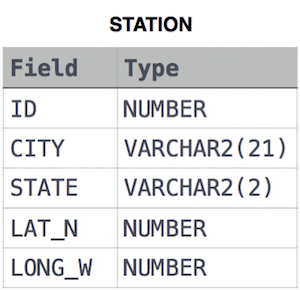
Where LAT_N is the northern latitude, and LONG_W is the western longitude.
To query the list of city names starting with vowels (a, e, i, o, or u) from the STATION
table, and ensure the result does not contain duplicates, you can use the following SQL query:
SELECT DISTINCT CITY
FROM STATION
WHERE CITY REGEXP '^[aeiouAEIOU]';
Here’s how the query works:
SELECT DISTINCT CITY
: This selects the unique city names from theSTATION
table.FROM STATION
: Specifies the table to query from.WHERE CITY REGEXP '^[aeiouAEIOU]'
: This condition filters the rows to include only those where theCITY
name starts with a vowel (either lowercase or uppercase) using the regular expression^[aeiouAEIOU]
.
This query will return the list of unique city names from the STATION
table that start with vowels without any duplicate
Query the list of # ending with vowels
Query the list of CITY names ending with vowels (a, e, i, o, u) from STATION. Your result cannot contain duplicates. The STATION table is described as follows:
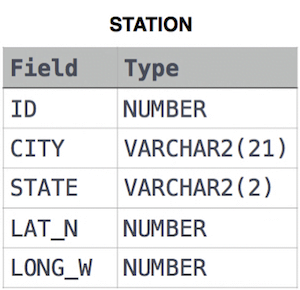
To query the list of city names ending with vowels (a, e, i, o, u) from the STATION
table, ensuring the result does not contain duplicates, you can use the following SQL query:
SELECT DISTINCT CITY
FROM STATION
WHERE CITY REGEXP '[aeiouAEIOU]$';
Here’s how the query works:
SELECT DISTINCT CITY
: This selects the unique city names from theSTATION
table.FROM STATION
: Specifies the table to query from.WHERE CITY REGEXP '[aeiouAEIOU]$'
: This condition filters the rows to include only those where theCITY
name ends with a vowel (either lowercase or uppercase) using the regular expression[aeiouAEIOU]$
.
This query will return the list of unique city names from the STATION
table that ends with vowels, without any duplicates.
Query the list of # starting & ending with vowels
Query the list of CITY names from STATION with vowels (i.e., a, e, i, o, and u) as their first and last characters. Your result cannot contain duplicates.
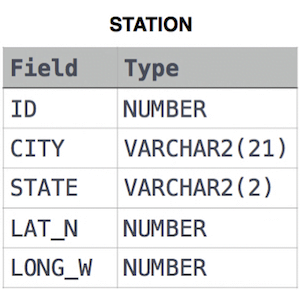
Query the list of city names from the STATION
the table which have vowels (a, e, i, o, and u) as both their first and last characters, ensuring the result does not contain duplicates, you can use the following SQL query:
SELECT DISTINCT CITY
FROM STATION
WHERE CITY REGEXP '^[aeiouAEIOU].*[aeiouAEIOU]$';
Here’s how the query works:
SELECT DISTINCT CITY
: This selects the unique city names from theSTATION
table.FROM STATION
: Specifies the table to query from.WHERE CITY REGEXP '^[aeiouAEIOU].*[aeiouAEIOU]$'
: This condition filters the rows to include only those where theCITY
name starts and ends with a vowel (either lowercase or uppercase) using the regular expression'^[aeiouAEIOU].*[aeiouAEIOU]$'
.
This query will return the list of unique city names from the STATION
table with vowels as their first and last characters without duplicates.
Query the list of # not starting with vowels
Query the list of CITY names from STATION that do not start with vowels. Your result cannot contain duplicates.
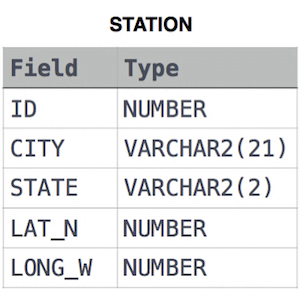
To query the list of city names from the STATION
table that do not begin with vowels, ensuring the result does not contain duplicates, you can use the following SQL query:
SELECT DISTINCT CITY
FROM STATION
WHERE CITY NOT REGEXP '^[aeiouAEIOU]';
Here’s how the query works:
SELECT DISTINCT CITY
: This selects the unique city names from theSTATION
table.FROM STATION
: Specifies the table to query from.WHERE CITY NOT REGEXP '^[aeiouAEIOU]'
: This condition filters the rows to include only those where theCITY
name does not start with a vowel (either lowercase or uppercase) using the regular expression'^[aeiouAEIOU]'
.
This query will return the list of unique city names from the STATION
table that does not start with vowels, without any duplicates.
Query the list of # not ending with vowels
Query the list of CITY names from STATION that do not end with vowels. Your result cannot contain duplicates.
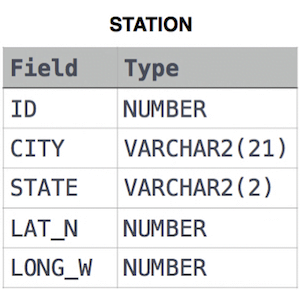
To query the list of city names from the STATION
table that do not end with vowels, ensuring the result does not contain duplicates, you can use the following SQL query:
SELECT DISTINCT CITY
FROM STATION
WHERE CITY NOT REGEXP '[aeiouAEIOU]$';
Here’s how the query works:
SELECT DISTINCT CITY
: This selects the unique city names from theSTATION
table.FROM STATION
: Specifies the table to query from.WHERE CITY NOT REGEXP '[aeiouAEIOU]$'
: This condition filters the rows to include only those where theCITY
name does not end with a vowel (either lowercase or uppercase) using the regular expression[aeiouAEIOU]$
.
This query will return the list of unique city names from the STATION
table that do not end with vowels, without any duplicates.
Query the list of # either not starting or not ending with vowels
Query the list of CITY names from STATION that either do not begin with vowels or do not end with vowels. Your result cannot contain duplicates.
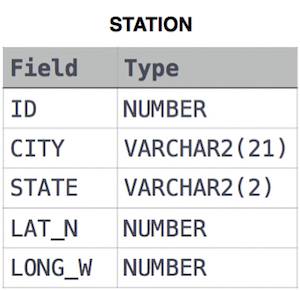
To query the list of city names from the STATION
table that either do not start with vowels or does not end with vowels, ensuring the result does not contain duplicates, you can use the following SQL query:
SELECT DISTINCT CITY
FROM STATION
WHERE CITY NOT REGEXP '^[aeiouAEIOU]' OR CITY NOT REGEXP '[aeiouAEIOU]$';
Here’s how the query works:
SELECT DISTINCT CITY
: This selects the unique city names from theSTATION
table.FROM STATION
: Specifies the table to query from.WHERE CITY NOT REGEXP '^[aeiouAEIOU]' OR CITY NOT REGEXP '[aeiouAEIOU]$'
: This condition filters the rows to include only those where theCITY
name does not start with vowels OR does not end with vowels.
This query will return the list of unique city names from the STATION
table that either do not start with vowels or do not end with vowels, without any duplicates.
Query the list of # that do not begin nor end with vowels
Query the list of CITY names from STATION that do not start with vowels or end with vowels. Your result cannot contain duplicates.
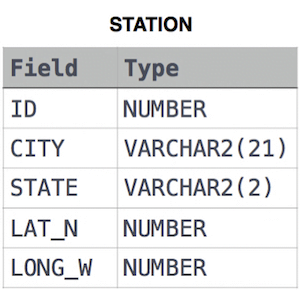
To query the list of city names from the STATION
table that do not start with vowels and do not end with vowels, ensuring the result does not contain duplicates, you can use the following SQL query:
SELECT DISTINCT CITY
FROM STATION
WHERE CITY NOT REGEXP '^[aeiouAEIOU]' AND CITY NOT REGEXP '[aeiouAEIOU]$';
Here’s how the query works:
SELECT DISTINCT CITY
: This selects the unique city names from theSTATION
table.FROM STATION
: Specifies the table to query from.WHERE CITY NOT REGEXP '^[aeiouAEIOU]' AND CITY NOT REGEXP '[aeiouAEIOU]$'
: This condition filters the rows to include only those where theCITY
name neither starts nor ends with vowels.
This query will return the list of unique city names from the STATION
table that does not start with vowels or end with vowels without duplicates.